
Computers and Technology, 23.01.2022 04:00 hollandhogenson
Project is due tonight - Please help!
I need to create a game in Java that involves the card game 21, and here is the files:
Main. java
import java. util.*; //for the Scanner class methods
class Main {
public static void main(String[] args){
Scanner input = new Scanner(System. in);
//declare 3 cards for you and 3 cards for the computer; call the dealCard method to generate a unique card as shown.
Card myC1 = dealCard();
Card myC2 = dealCard();
Card myC3 = dealCard();
Card botC1 = dealCard();
Card botC2 = dealCard();
Card botC3 = dealCard();
//print your 2 Cards & total
System. out. println(myC1);
System. out. println(myC2);
System. out. println("Your cards: " + myC1 + ", " + myC2);
//if you have 21, you win, game ends.
if((myC1 + myC2) == 21){
System. out. println("You have won the game!");
}
//print the computers first card
System. out. println(botC1);
System. out. println(botC2);
System. out. println("Bot's cards: " + botC1 + ", " + botC2);
//ask if user wants another card & print out those 3 cards & total if so
String promptCont = input. nextLine();
System. out. println("Would you like to add another card?");
if (promptCont == "y"){
System. out. println(myC1);
System. out. println(myC2);
System. out. println(myC3);
}
//give computer another card if computer total < 17
if((botC1 + botC2) < 17){
System. out. println(botC1);
System. out. println(botC2);
System. out. println(botC3);
}
//print out computer's cards & totals
System. out. println(botC1 + botC2 + botC3);
//determine and print the winner
if((botC1 + botC2 + botC3) == 21){
System. out. println("Bot wins!");
}
else if((botC1 + botC2 + botC3) + (myC1 + myC2 + myC3) != 21){
System. out. println("nobody won :(");
} else if((myC1 + myC2 + myC3) == 21){
System. out. println("You win!");
}
}//end of main method
//don't change this method
public static Card dealCard(){
Card temp = new Card();
String[] names = {"Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King", "Ace"};
int num = (int)(Math. random()*52);
while(Card. getDeck()[num]==true)
num = (int)(Math. random()*52 );
switch(num/13){
case 0: temp = new Card(names[num%13], "Clubs", num+2 ); break;
case 1: temp = new Card(names[num%13], "Diamonds", num+2-13); break;
case 2: temp = new Card(names[num%13], "Hearts", num+2-26); break;
case 3: temp = new Card(names[num%13], "Spades", num+2-39); break;
}
if(temp. getPointValue()==11 || temp. getPointValue()==12 || temp. getPointValue()==13)
temp. setPointValue(10); //in 21, face cards = 10
if(temp. getPointValue()==14)
temp. setPointValue(11); //in 21, aces = 11
Card. addToDeck(temp);
return temp;
} //end of dealCard method
} //end of Main. java class
Card. java
//Card class goes here.
public class Card{
private String rank;
private String suit;
private int PointValue;
private static boolean[] deck = new boolean[52]; //instance field
public void initDeck()
{
this. rank = "None";
this. suit = "None";
this. PointValue = 0;
}
public void Cards(String rank, String suit, int PointValue)
{
this. rank = rank;
this. suit = suit;
this. PointValue = PointValue;
}
//these methods are part of the Card class.
public static boolean[] getDeck(){
return deck;
}
public static int[] getPointValue(){
PointValue = this. PointValue;
return PointValue;
}
public static void setPointValue(int newVal){
}
public static void addToDeck(Card c1)
{
if(c1.suit. equals("Clubs"))
deck[c1.pointValue - 2] = true;
else if (c1.suit. equals("Diamonds"))
deck[c1.pointValue - 2 + 13] = true;
else if (c1.suit. equals("Hearts"))
deck[c1.pointValue - 2 + 26] = true;
else
deck[c1.pointValue - 2 + 39] = true;
}
}
The rubric is attached. Thanks yall :)

Answers: 1
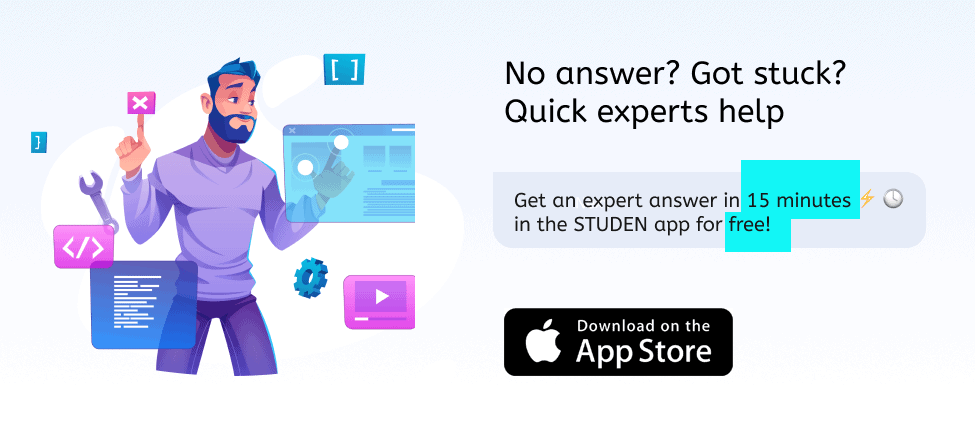

Another question on Computers and Technology

Computers and Technology, 22.06.2019 04:50
Which are steps taken to diagnose a computer problem? a) reproducing the problem and using error codes b) reproducing the problem and troubleshooting c) using error codes and troubleshooting d) using error codes and stepping functions
Answers: 1

Computers and Technology, 23.06.2019 01:30
Jason works as an accountant in a department store. he needs to keep a daily record of all the invoices issued by the store. which file naming convention would him the most? a)give the file a unique name b)name the file in yymmdd format c)use descriptive name while naming the files d)use capital letters while naming the file
Answers: 3

Computers and Technology, 23.06.2019 15:20
In a game with three frames, where will the objects on layer 1 appear? a. next to the play area b. in the middle of the game c. behind everything else d. in front of everything else
Answers: 1

Computers and Technology, 23.06.2019 16:00
An english teacher would like to divide 8 boys and 10 girls into groups, each with the same combination of boys and girls and nobody left out. what is the greatest number of groups that can be formed?
Answers: 2
You know the right answer?
Project is due tonight - Please help!
I need to create a game in Java that involves the card game...
Questions

Mathematics, 29.07.2021 06:50

Advanced Placement (AP), 29.07.2021 07:20

History, 29.07.2021 07:20

Biology, 29.07.2021 07:20


Social Studies, 29.07.2021 07:20

Mathematics, 29.07.2021 07:20

Mathematics, 29.07.2021 07:20

English, 29.07.2021 07:20

Mathematics, 29.07.2021 07:20

History, 29.07.2021 07:20




Biology, 29.07.2021 07:20




English, 29.07.2021 07:20

Chemistry, 29.07.2021 07:20