
Computers and Technology, 24.11.2021 19:40 bdog2213
Must be in java and have testers
b) Shift all elements by one to the right and move the last element into the first position. For example,
1 4 9 16 25 would become 25 1 4 9 16.
c) Replace all even elements with 0.
d) Replace each element except the first and last by the larger of its two neighbors. For the example in
part (b), your output would be 1 9 16 25 25.
e) Remove the middle element if the array length is odd, or the middle two elements if the length is
even. (You will need to test two different arrays here to make sure your method works on both even
and odd length arrays).
f) Move all even elements to the front, otherwise preserving the order of the elements. For the example
in part (b) your output should be 4 16 1 9 25
g) Return the second-largest element in the array. (Your method should return this value, and your
main method should print the value out).
h) Return true if the array is currently sorted in increasing order. (You can use Arrays. sort() to sort an
array before you call your method for testing purposes. Make sure to test both a sorted array and an
unsorted array as input). Your main method should print whether the array was in order or not.
i) Return true if the array contains two adjacent duplicate elements.
j) Return true if the array contains duplicate elements (which need not be adjacent).
starting code:
import java. util. Arrays;
import java. util. Random;
public class ArrayMethods {
//***NOTE that these methods will change the array itself
//part a, fill in this method
public static void swapFirstAndLast(int[] values) {
// save the first element to a temp var
int temp = values[0];
//move the last element to the first position
values[0] = values[values. length-1];
// now put the saved first element into the last position
values[values. length-1] = temp;
}
//part b, fill in this method
public static void shiftRight(int[] values) {
}
//part c, set all even elements to 0.
public static void setEvensToZero(int[] values) {
}
//part d, replace each element except the first and last by larger of two
//around it
public static void largerOfAdjacents(int[] values) {
}
//part e, remove middle el if odd length, else remove middle two els.
public static int[] removeMiddle(int[] values) {
//replace the following line with your answer
//this line needed to compile
return null;
}
//part f - move all evens to front
public static void moveEvensToFront(int[] values) {
}
//part g - return second largest element in array
public static int ret2ndLargest(int[] values) {
// replace this line with your correct return value
return 0;
}
//part H - returns true if array is sorted in increasing order
public static boolean isSorted(int[] values) {
// replace this line with your correct return value
return false;
}
//PART I - return true if array contains 2 adjacent duplicate values
public static boolean hasAdjDuplicates(int[] values) {
// replace this line with your correct return value
return false; //dummy return value
}
//PART J - return true if array contains 2 duplicate values
//duplicates need not be adjacent to return true
public static boolean hasDuplicates(int[] values) {
// replace this line with your correct return value
return false;
}
}
tester code:
import java. util. Arrays;
import java. util. Random;
public class ArrayMethodsTester {
//helper method to print an array
public static void printArray(int[] values) {
System. out. println(Arrays. toString(values));
}
public static void main(String[] args) {
//In your main method you should test your array methods
//Create an array of size 10
// HERE
int[] a = new int[1]; //array of size 1
// Fill the array with random values (use a loop, and a
//Random object)
//Now print the array to show initial values
System. out. println("Initial Array:");
//note the usage of the "toString()" method here to print the array
System. out. println(Arrays. toString(a));
//Could replace the previous line with this:
//printArray(testValues);
//blank line
System. out. println();
//Test methods below this line.
//Test of swapFirstAndLast()
System. out. println("Before call to swapFirstAndLast():");
printArray(a);
//swap first and last element
//this method modifies the array referenced by "testValues"
ArrayMethods. swapFirstAndLast(a);
System. out. println("After call to swapFirstAndLast()");
printArray(a); //printing the same array but it has changed
System. out. println();
//continue with tests as you complete methods ... }
}

Answers: 3
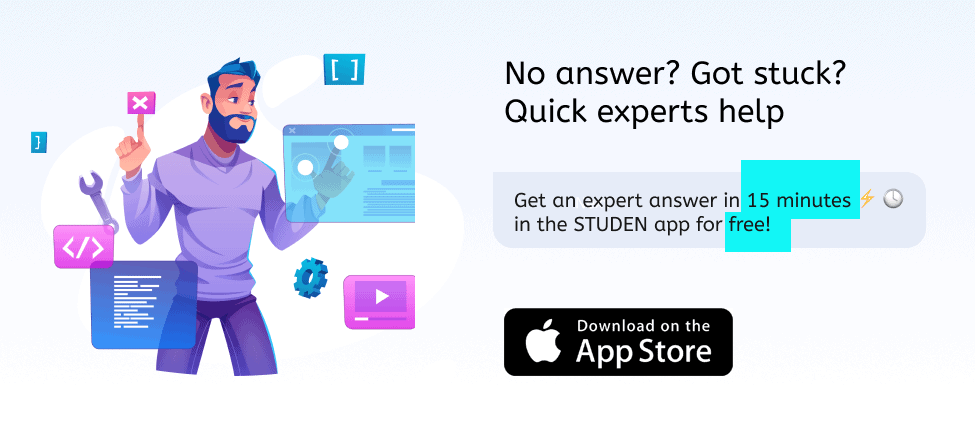

Another question on Computers and Technology

Computers and Technology, 21.06.2019 19:20
Number of megabytes of ram in a computer. qualitative or quantitative? because
Answers: 3

Computers and Technology, 22.06.2019 20:00
The blank button automatically displays next to the data when you select a range of numeric data which is an available option for creating a chart
Answers: 3

Computers and Technology, 23.06.2019 03:50
Q-1 which of the following can exist as cloud-based it resources? a. physical serverb. virtual serverc. software programd. network device
Answers: 1

Computers and Technology, 23.06.2019 09:30
You wanted to look up information about alzheimer's, but you were unsure if it was spelled "alsheimer's" or "alzheimer's." which advanced search strategy would be useful? a) a boolean search b) using a wild card in your search c) trying different search engines d) doing a search for "alsheimer's not alzheimer's" asap. ill give brainlist.
Answers: 1
You know the right answer?
Must be in java and have testers
b) Shift all elements by one to the right and move the last eleme...
Questions



English, 29.01.2021 01:00

Mathematics, 29.01.2021 01:00


English, 29.01.2021 01:00



Mathematics, 29.01.2021 01:00



Mathematics, 29.01.2021 01:00

Biology, 29.01.2021 01:00

Mathematics, 29.01.2021 01:00

English, 29.01.2021 01:00





English, 29.01.2021 01:00