
Computers and Technology, 24.05.2021 15:50 joejoefofana
Zip code and population (generic types) Define a class StatePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printInfo() method. Three ArrayLists have been pre-filled with StatePair data in main(): ArrayList> zipCodeState: Contains ZIP code/state abbreviation pairs ArrayList> abbrevState: Contains state abbreviation/state name pairs ArrayList> statePopulation: Contains state name/population pairs After a ZIP code is read into the program, complete main() to retrieve the correct state abbreviation from the ArrayList zipCodeState. Then use the state abbreviation to retrieve the state name from the ArrayList abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the ArrayList statePopulation and output the pair. Ex: If the input is 21044 the output is Maryland: 6079602
Code already written:
StatePopulations. java
import java. util. Scanner;
import java. io. FileInputStream;
import java. io. IOException;
import java. util. ArrayList;
public class StatePopulations {
public static ArrayList> fillArray1(ArrayList> statePairs,
Scanner inFS) {
StatePair pair;
int intValue;
String stringValue;
while (inFS. hasNextLine()) {
intValue = inFS. nextInt();
stringValue = inFS. next();
pair = new StatePair (intValue, stringValue);
statePairs. add(pair);
}
return statePairs;
}
public static ArrayList> fillArray2(ArrayList> statePairs,
Scanner inFS) {
StatePair pair;
String stringValue1;
String stringValue2;
while (inFS. hasNextLine()) {
stringValue1 = inFS. next();
inFS. nextLine();
stringValue2 = inFS. nextLine();
pair = new StatePair (stringValue1, stringValue2);
statePairs. add(pair);
}
return statePairs;
}
public static ArrayList> fillArray3(ArrayList> statePairs,
Scanner inFS) {
StatePair pair;
String stringValue;
int intValue;
while (inFS. hasNextLine()) {
stringValue = inFS. nextLine();
intValue = inFS. nextInt();
inFS. nextLine();
pair = new StatePair (stringValue, intValue);
statePairs. add(pair);
}
return statePairs;
}
public static void main(String[] args) throws IOException {
Scanner scnr = new Scanner(System. in);
FileInputStream fileByteStream = null; // File input stream
Scanner inFS = null; // Scanner object
int myZipCode;
int i;
// ZIP code - state abbrev. pairs
ArrayList> zipCodeState = new ArrayList>();
// state abbrev. - state name pairs
ArrayList> abbrevState = new ArrayList>();
// state name - population pairs
ArrayList> statePopulation = new ArrayList>();
// Fill the three ArrayLists
// Try to open zip_code_state. txt file
fileByteStream = new FileInputStream("zip_code_state. txt");
inFS = new Scanner(fileByteStream);
zipCodeState = fillArray1(zipCodeState, inFS);
fileByteStream. close(); // close() may throw IOException if fails
// Try to open abbreviation_state. txt file
fileByteStream = new FileInputStream("abbreviation_state . txt");
inFS = new Scanner(fileByteStream);
abbrevState = fillArray2(abbrevState, inFS);
fileByteStream. close(); // close() may throw IOException if fails
// Try to open state_population. txt file
fileByteStream = new FileInputStream("state_population. txt");
inFS = new Scanner(fileByteStream);
statePopulation = fillArray3(statePopulation, inFS);
fileByteStream. close(); // close() may throw IOException if fails
// Read in ZIP code from user
myZipCode = scnr. nextInt();
for (i = 0; i < zipCodeState. size(); ++i) {
// TODO: Using ZIP code, find state abbreviation
}
for (i = 0; i < abbrevState. size(); ++i) {
// TODO: Using state abbreviation, find state name
}
for (i = 0; i < statePopulation. size(); ++i) {
// TODO: Using state name, find population. Print pair info.
}
}
}
StatePair. java
public class StatePair , Type2 extends Comparable> {
private Type1 value1;
private Type2 value2;
// TODO: Define a constructor, mutators, and accessors
// for StatePair
// TODO: Define printInfo() method
}

Answers: 2
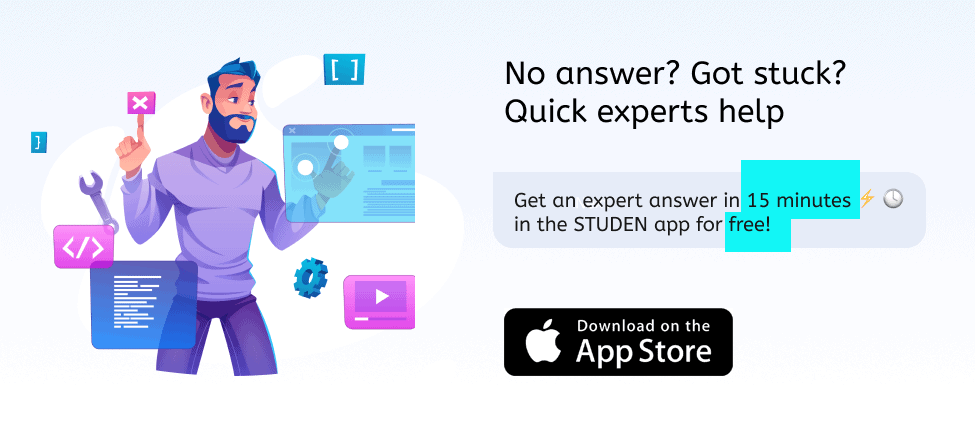

Another question on Computers and Technology

Computers and Technology, 23.06.2019 02:50
Define a class named movie. include private fields for the title,year, and name of the director. include three public functions withprototypes void movie: : settitle(cstring); , voidmovie: : setyear(int); , void movie: : setdirector(string); . includeanother function that displays all the information about a movie.write a main() function that declares a movie object namedmyfavoritemovie. set and display the object's fields.this is what i have but know its wrong since it will notcompile: #include#includeusing namespace std; //class declarationclass movie{private: string movietitle ; string movieyear; string directorname; public: void settitle(string title); void setyear(string year); void setdirector(string director); void displayinfo(); }; //class implementationvoid movie: : settitle(string title){ movietitle = title; cout< < "what is the title of themovie? "< > temp; myfavoritemovie.settitle(temp); cout< < "enter movie year"< > temp; myfavoritemovie.setyear(temp); cout< < "enter director'sname"< > temp; myfavoritemovie.setdirector(temp); //display all the data myfavoritemovie.displayinfo(); system("pause"); return 0; this code is not entirely mine someone on cramster edited my firstcode but then i try manipulating the new code and i still get acompile error message : \documents\visual studio 2008\projects\movie\movie\movie.cpp(46) : error c2679: binary '< < ' : no operator found which takes aright-hand operand of type 'std: : string' (or there is no acceptableconversion)c: \program files (x86)\microsoft visual studio9.0\vc\include\ostream(653): could be'std: : basic_ostream< _elem,_traits> & std: : operator< < > (std: : basic_ostream< _elem,_traits> & ,const char *)w
Answers: 1

Computers and Technology, 24.06.2019 00:50
Which player type acts on other players? a. killer b. achiever c. explorer d. socializer
Answers: 1

Computers and Technology, 24.06.2019 02:30
How to apply the fly in effect to objects on a slide
Answers: 1

Computers and Technology, 24.06.2019 11:30
Convert 11001110(acdd notation) into decimal
Answers: 2
You know the right answer?
Zip code and population (generic types) Define a class StatePair with two generic types (Type1 and T...
Questions



Mathematics, 08.06.2020 09:57


Health, 08.06.2020 09:57

English, 08.06.2020 09:57

Law, 08.06.2020 09:57



Mathematics, 08.06.2020 09:57

Arts, 08.06.2020 09:57

Social Studies, 08.06.2020 09:57

Mathematics, 08.06.2020 09:57


History, 08.06.2020 09:57



English, 08.06.2020 09:57

Mathematics, 08.06.2020 09:57