
Computers and Technology, 24.05.2021 15:20 Kurlyash
The program below represents decimal integers as a linked list of digits. It asks for 2 positive integers, stores them as linked lists, computes the sum, and outputs it. It then freeO's all nodes of all 3 lists. a. You must use my struct Digi tNode b. I strongly suggest that you represent the integers in little-endian order. That will make add 0) way easier. Please write: obtainPostiveInt 。numberList () o add printList o freeList Sample output: (No surprises here, it is just decimal integer addition, but these are some interesting boundary cases to make sure you handle.)
C CODE (COPY/PASTE):
/**
*--- ---*
*--- byDigitAdder. c ---*
*--- ---*
*--- This file defines a C program that represents positive ---*
*--- decimal integers as linked lists of digits. It asks the user ---*
*--- for 2 integers, computes the sum, and outputs it. ---*
*--- ---*
*-*
*--- ---*
*--- Version 1a 2018 February 11 Joseph Phillips ---*
*--- ---*
**/
#include
#include
#include
#define NUM_TEXT_LEN 256// PURPOSE: To represent one digit of a decimal number.
struct DigitNode
{
int digit_; // I suggest you range this in [0..9]
struct DigitNode* nextPtr_; // I suggest you make this point to
// the next most significant digit.
};
// PURPOSE: To obtain the text of a decimal integer into array 'numberCPtr'
// of length 'numberTextLen'. 'descriptionCPtr' is printed because it
// tells the user the integer that is expected. Ending '\n' from
// 'fgets()' is replaced with '\0'. No return value.
void obtainPostiveInt(char* numberCPtr,
int numberTextLen,
const char* descriptionCPtr
)
{
// YOUR CODE HERE
}
// PURPOSE: To build and return a linked list IN LITTLE ENDIAN ORDER
// of the decimal number whose text is pointed to by 'numberCPtr'.
// If 'numberCPtr' points to the string "123" then the linked list
// returned is 'digit_=3' -> 'digit_=2' -> 'digit_=1' -> NULL.
struct DigitNode*
numberList (const char* numberCPtr
)
{
// YOUR CODE HERE
}
// PURPOSE: To build and return a linked list IN LITTLE ENDIAN ORDER
// of the decimal number that results from adding the decimal numbers
// whose digits are pointed to by 'list0' and 'list1'.
struct DigitNode*
add (const struct DigitNode* list0,
const struct DigitNode* list1
)
{
// YOUR CODE HERE
}
// PURPOSE: To print the decimal number whose digits are pointed to by 'list'.
// Note that the digits are IN LITTLE ENDIAN ORDER. No return value.
void printList (const struct DigitNode* list
)
{
// YOUR CODE HERE
}
// PURPOSE: To print the nodes of 'list'. No return value.
void freeList (struct DigitNode* list
)
{
// YOUR CODE HERE
}
// PURPOSE: To coordinate the running of the program. Ignores command line
// arguments. Returns 'EXIT_SUCCESS' to OS.
int main ()
{
char numberText0[NUM_TEXT_LEN];
char numberText1[NUM_TEXT_LEN];
struct DigitNode* operand0List = NULL;
struct DigitNode* operand1List = NULL;
struct DigitNode* sumList = NULL;
obtainPostiveInt(numberText0,NUM_TE XT_LEN,"first");
obtainPostiveInt(numberText1,NUM_TE XT_LEN,"second");
operand0List = numberList(numberText0);
operand1List = numberList(numberText1);
sumList = add(operand0List, operand1List);
printList(operand0List);
printf(" + ");
printList(operand1List);
printf(" = ");
printList(sumList);
printf("\n");
freeList(sumList);
freeList(operand1List);
freeList(operand0List);
return(EXIT_SUCCESS);
}

Answers: 1
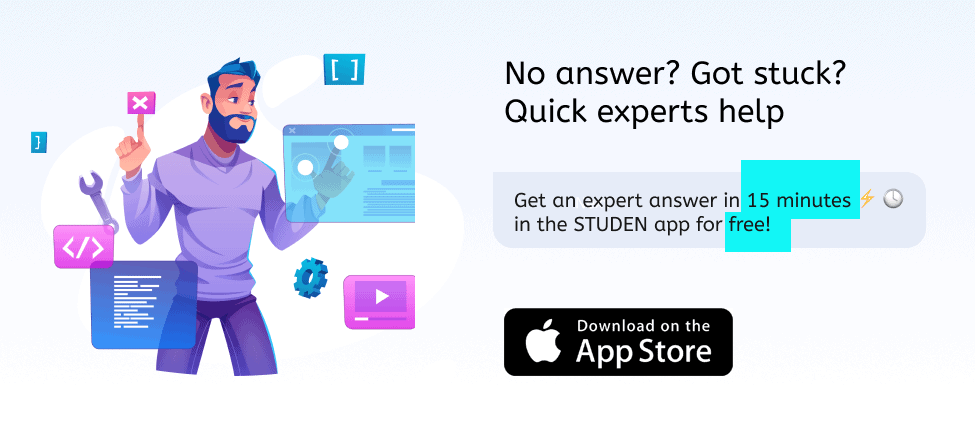

Another question on Computers and Technology

Computers and Technology, 22.06.2019 02:30
If you turn on the lock alpha button , what happens
Answers: 1

Computers and Technology, 23.06.2019 09:00
Which best describes the role or restriction enzymes in the analysis of edna a. to break dna into fragments that vary in size so they can be sorted and analyzed b. to amplify small amounts of dna and generate large amounts of dna for analysis c. to purify samples of dna obtained from the environment so they can be analyzed d. to sort different sizes of dna fragments into a banding pattern that can be analyzed
Answers: 1

Computers and Technology, 23.06.2019 19:30
Anul 2017 tocmai s-a încheiat, suntem trişti deoarece era număr prim, însă avem şi o veste bună, anul 2018 este produs de două numere prime, 2 şi 1009. dorel, un adevărat colecţionar de numere prime, şi-a pus întrebarea: “câte numere dintr-un interval [a,b] se pot scrie ca produs de două numere prime? “.
Answers: 3

Computers and Technology, 24.06.2019 06:30
Some peer-to-peer networks have a server and some don't. true false
Answers: 2
You know the right answer?
The program below represents decimal integers as a linked list of digits. It asks for 2 positive int...
Questions








History, 28.08.2019 05:20


Mathematics, 28.08.2019 05:20


Mathematics, 28.08.2019 05:20

Mathematics, 28.08.2019 05:20




Mathematics, 28.08.2019 05:20



Mathematics, 28.08.2019 05:20