Extend by inheritance the BankAccount Class to create two new subclasses:
SavingsAccount
Che...

Computers and Technology, 04.05.2021 21:20 cesargonzaleztovar60
Extend by inheritance the BankAccount Class to create two new subclasses:
SavingsAccount
CheckingAccount
DO NOT CODE ANY METHODS IN THE SUBCLASSES OTHER THAN THE CONSTRUCTOR. ALL THE METHODS SHOULD COME FROM THE PARENT CLASS AUTOMATICALLY BY INHERITANCE.
CODING METHODS (OTHER THAN THE CONSTRUCTOR) IN THE SUBCLASSES WILL VOID YOUR LAB FROM ANY CREDIT.
Your BankAccount is the parent class.
Be sure to ADD to your BankAccount class the static method transfer(BankAccount origin, BankAccount destination) from Exam 2.
If you did not code this method in your exam 2, I will post one in this week's discussion.
In main(), use the file accounts-1.txt create an array of BankAccount, CheckingAccount and SavingsAccount objects.
This can be done using the following fragment in main():
// create the arrays of the 3 types of accounts
BankAccount[] accounts = new BankAccount[fileSize];
SavingAccount[] savingsAccounts = new SavingsAccount[fileSize];
CheckingAccount[] checkingAccounts = new CheckingAccount[fileSize];
// Use a loop to call the constructors
for(int i=0 ; i 0)
{
System. out. println("The account was located in record "+ location);
System. out. println(accounts[location]);
System. out. println("Checking:\n " + checkingAccounts[location]);
System. out. println("Savings:\n "+ savingsAccounts[location]);
}
else
{
System. out. println("No account with id " +id + " was located");
}
Now, if you run the sample code from above, and the accounts were properly constructed, we expect the following result:
Please enter the account ID: 55922
The account was located in record 950
Account #: 55922
Owner: Doyle May
Balance: $4,355.59
Checking:
Account #: 55922
Owner: Doyle May
Balance: $2,177.80
Savings:
Account #: 55922
Owner: Doyle May
Balance: $2,177.80
The last step for this Lab is to use the static method from Exam 2
BankAccount. transfer(BankAccount origin, BankAccount destiny)
to transfer all the money from the savings account to the checking account. Using the same account from the example above, we can code the transfer in main() like follows:
BankAccount. transfer( savingsAccounts[location], checkingAccounts[location] );
System. out. println(accounts[location]);
System. out. println("Checking after transfer:\n " + checkingAccounts[location]);
System. out. println("Savings after transfer:\n "+ savingsAccounts[location]);
The output if the method is properly working should be:
Account #: 55922
Owner: Doyle May
Balance: $4,355.59
Checking after transfer:
Account #: 55922
Owner: Doyle May
Balance: $4,355.59
Savings after transfer:
Account #: 55922
Owner: Doyle May
Balance: $0.00
As you can see, the balances were updated by the transfer method.
SUBMIT THREE (3) files: The file with your main() method, the subclasses SavingsAccount. java and CheckingAccount. java
(The files needed for the code are attached the BankAccount java is suppose to the super class while, the accounts-1 text file is what you have to incorporate with it)

Answers: 3
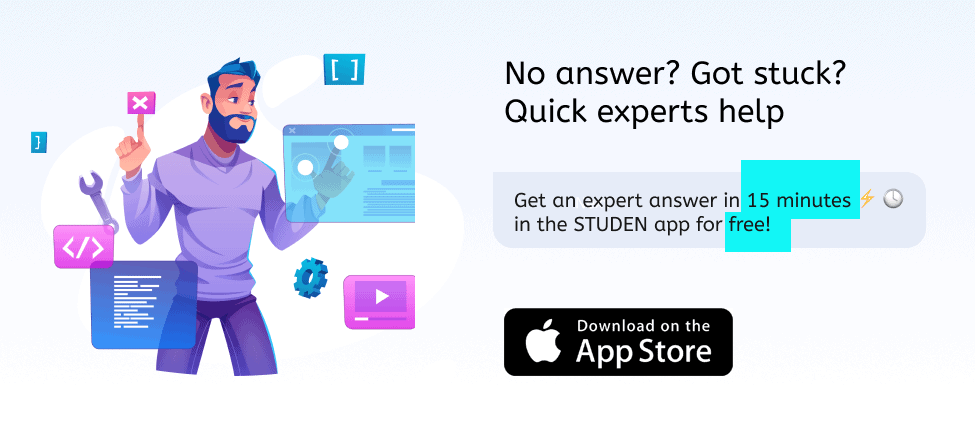

Another question on Computers and Technology

Computers and Technology, 22.06.2019 15:30
When creating a budget, log fixed expenses before income. after income. after savings. at the top.
Answers: 1

Computers and Technology, 23.06.2019 02:00
In the context of an internet connection, llc stands for leased line connection liability limited company local loop complex local loop carrier
Answers: 1

Computers and Technology, 23.06.2019 18:00
What can a word user do with the customize ribbon dialog box? check all that apply. minimize the ribbon add a new tab to the ribbon remove a group from a tab add a group to a tab choose which styles appear choose which fonts appear choose tools to appear in a group
Answers: 1

Computers and Technology, 24.06.2019 03:00
With editing, word automatically displays a paste options button near the pasted or moved text. a. cut-and-paste b. drag-and-drop c. inline d. copy-and-carry
Answers: 1
You know the right answer?
Questions

Mathematics, 02.04.2020 17:44

English, 02.04.2020 17:44

Geography, 02.04.2020 17:44




Mathematics, 02.04.2020 17:45


History, 02.04.2020 17:45


Geography, 02.04.2020 17:45






Mathematics, 02.04.2020 17:45

Biology, 02.04.2020 17:46
