
Computers and Technology, 23.04.2021 16:00 andy2461
In Scheme, source code is data. Every non-atomic expression is written as a Scheme list, so we can write procedures that manipulate other programs just as we write procedures that manipulate lists.
Rewriting programs can be useful: we can write an interpreter that only handles a small core of the language, and then write a procedure that converts other special forms into the core language before a program is passed to the interpreter.
For example, the let special form is equivalent to a call expression that begins with a lambda expression. Both create a new frame extending the current environment and evaluate a body within that new environment. Feel free to revisit Problem 15 as a refresher on how the let form works.
(let ((a 1) (b 2)) (+ a b))
;; Is equivalent to:
((lambda (a b) (+ a b)) 1 2)
These expressions can be represented by the following diagrams:
Let Lambda
let lambda
Use this rule to implement a procedure called let-to-lambda that rewrites all let special forms into lambda expressions. If we quote a let expression and pass it into this procedure, an equivalent lambda expression should be returned: pass it into this procedure:
scm> (let-to-lambda '(let ((a 1) (b 2)) (+ a b)))
((lambda (a b) (+ a b)) 1 2)
scm> (let-to-lambda '(let ((a 1)) (let ((b a)) b)))
((lambda (a) ((lambda (b) b) a)) 1)
In order to handle all programs, let-to-lambda must be aware of Scheme syntax. Since Scheme expressions are recursively nested, let-to-lambda must also be recursive. In fact, the structure of let-to-lambda is somewhat similar to that of scheme_eval--but in Scheme! As a reminder, atoms include numbers, booleans, nil, and symbols. You do not need to consider code that contains quasiquotation for this problem.
(define (let-to-lambda expr)
(cond ((atom? expr) )
((quoted? expr) )
((lambda? expr) )
((define? expr) )
((let? expr) )
(else )))
CODE:
; Returns a function that checks if an expression is the special form FORM
(define (check-special form)
(lambda (expr) (equal? form (car expr
(define lambda? (check-special 'lambda))
(define define? (check-special 'define))
(define quoted? (check-special 'quote))
(define let? (check-special 'let))
;; Converts all let special forms in EXPR into equivalent forms using lambda
(define (let-to-lambda expr)
(cond ((atom? expr)
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
)
((quoted? expr)
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
)
((or (lambda? expr)
(define? expr))
(let ((form (car expr))
(params (cadr expr))
(body (cddr expr)))
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
))
((let? expr)
(let ((values (cadr expr))
(body (cddr expr)))
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
))
(else
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
)))

Answers: 3
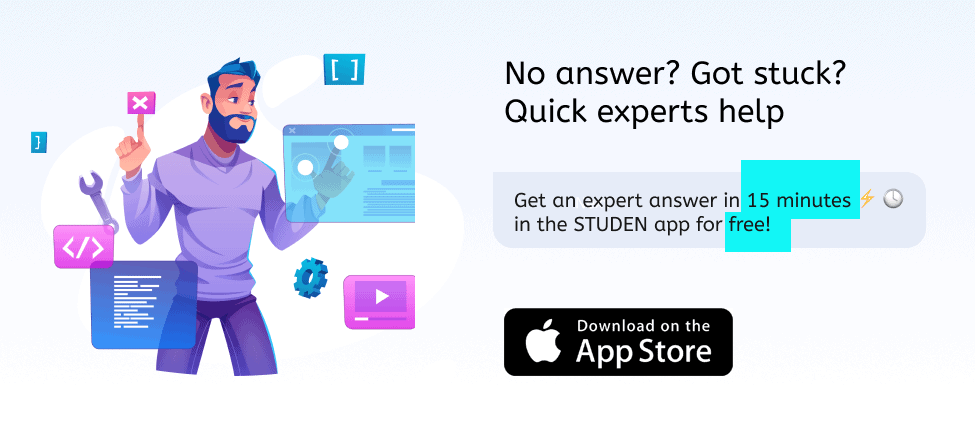

Another question on Computers and Technology

Computers and Technology, 22.06.2019 05:20
Write a program called assignment3 (saved in a ļ¬le assignment3.java) that computes the greatest common divisor of two given integers. one of the oldest numerical algorithms was described by the greek mathematician, euclid, in 300 b.c. it is a simple but very eāµective algorithm that computes the greatest common divisor of two given integers. for instance, given integers 24 and 18, the greatest common divisor is 6, because 6 is the largest integer that divides evenly into both 24 and 18. we will denote the greatest common divisor of x and y as gcd(x, y). the algorithm is based on the clever idea that the gcd(x, y) = gcd(x ! y, y) if x > = y and gcd(x, y) = gcd(x, y ! x) if x < y. the algorithm consists of a series of steps (loop iterations) where the ālargerā integer is replaced by the diāµerence of the larger and smaller integer. this continues until the two values are equal. that is then the gcd.
Answers: 3

Computers and Technology, 22.06.2019 11:00
Which are examples of note-taking tools? check all that recording devices sticky notes digital highlighters paper flags highlighting pens digital displays digital flags
Answers: 1

Computers and Technology, 22.06.2019 13:00
Write a program which asks you to enter a name in the form of first middle initial last. so you might enter for example samuel p. clemens. use getline to read in the string because it contains spaces. also, apparently the shift key on your keyboard doesnāt work, because you enter it all lower case. pass the string to a function which uses .find to locate the letters which need to be upper case and use toupper to convert those characters to uppercase. the revised string should then be returned to main in the form last, first mi where it will be displayed.
Answers: 1

Computers and Technology, 22.06.2019 22:40
Write a program that defines symbolic names for several string literals (chars between quotes). * use each symbolic name in a variable definition. * use of symbolic to compose the assembly code instruction set can perform vara = (vara - varb) + (varc - vard); ensure that variable is in unsigned integer data type. * you should also further enhance your symbolic logic block to to perform expression by introducing addition substitution rule. vara = (vara+varb) - (varc+vard). required: debug the disassembly code and note down the address and memory information.
Answers: 3
You know the right answer?
In Scheme, source code is data. Every non-atomic expression is written as a Scheme list, so we can w...
Questions




World Languages, 18.03.2021 01:20

Mathematics, 18.03.2021 01:20





Mathematics, 18.03.2021 01:20

Computers and Technology, 18.03.2021 01:20

Mathematics, 18.03.2021 01:20

English, 18.03.2021 01:20

Mathematics, 18.03.2021 01:20


Mathematics, 18.03.2021 01:20



German, 18.03.2021 01:20

Chemistry, 18.03.2021 01:20