
Computers and Technology, 23.04.2021 15:50 leshayk91
A terminal program allows you to communicate with the operating system through a series of commands. In this project, you are going to write a very simple terminal program that is capable of understanding the following commands: - ls : list the files and directories in the current working directory - cd : change the working directory to the directory given in the path argument. - pwd : prints the current working directory - mkdir : creates a new directory inside the current working directory - rm : removes the file given in the path - exit : causes the terminal to terminate. The project will be implemented via functions. But you need to learn about some of C system library functions first. Include the following libraries in your program: , ,
and Library functions:
1. S_ISDIR() : This function allows you to check whether a given path is a directory or not. It returns either true or false. struct stat buf; //data structure that holds file status lstat("/home/ubuntu/opencv. zip", &buf); // fills buffer about the argument path if(S_ISDIR(buf. st_mode)) // checks if path is a directory cout << "It is a directory" << endl; Similarly S_ISREG() returns true if the path is a file.
2. getcwd() : This function returns the current working directory in the form of a C string which is a char * cout << getcwd(NULL, 0) << endl; 3. Listing files inside a directory can be done via two functions: opendir() and readdir() DIR * dp; //directory data structure struct dirent * dirp; //directory entry data structure dp = opendir("/home/ubuntu"); //opens the directory for the given path while((dirp = readdir(dp)) != NULL) //reads directory entries one by one in dirp data structurecout d_name << endl;
4. c_str() : The functions introduced above accept C-strings as arguments. To convert a regular string variable into a C-string, we can call the c_str() function on the string object. The first sample program can be altered as follows: struct stat buf; //data structure that holds file status string path = "/home/ubuntu/opencv. zip"; lstat(path. c_str(), &buf); // fills the data structure about the argument path if(S_ISDIR(buf. st_mode)) // checks if path is a directory cout << "It is a directory" << endl;
5. mkdir(): Creates a new directory. int status; status = mkdir("newdir", S_IRWXU | S_IRWXG | S_IROTH | S_IXOTH); The function creates a directory named "newdir" under the current working directory using read-write-execute privileges for user and group and read-execute privileges for others.
6. chdir(): changes the current working directory to a different one. if(chdir("/home/ubuntu")<0) cout << "Coud not open path: " << path; 7. remove(): deletes the file or directory given a certain path. remove("/home/ubuntu/sample. txt"); Step1: Define and implement a function named isFile() based on the following prototype:
bool isFile(string path); The function will return true if the given path is a regular file and return false otherwise.
Step2: Define and implement a function named listFiles() based on the following prototype: void listFiles(string dirPath); The function will print all the files and directories under dirPath directory. You may assume that dirPath is a directory.
Step3: Define and implement a function named getCurrentDirectory() based on the following prototype: char * getCurrentDirectory(); This function does not take any parameter but returns the current working directory in the form of a C-string.
Step4. Define and implement a function named removeFile() based on the following prototype: void removeFile(string path); The function must check first whether the path is a file and then remove the file. Otherwise, the function prints a message saying "Cannot remove, it is not a file."
Step5: Write a main function which will call the functions above to implement a simple terminal program. The terminal program will accept commands from the user and act based
on the command type.
Sample output: ~/Spring2021/CS101/project2/ $ ./terminal myterminal>ls samples. cpp samples . terminal terminal. cpp .. myterminal>pwd /home/ubuntu/Spring2021/CS101/proje ct2 myterminal>cd .. /home/ubuntu/Spring2021/CS101 myterminal>ls Lab1 Lecture2 Lecture1 Lecture9 Lab3 . Lecture6 Lab2 Lecture3 Lab4 project2
mockexam1 .. Lecture7 myterminal>rm Lecture9/math myterminal>cd Lecture9/ /home/ubuntu/Spring2021/CS101/Lectu re9 myterminal>ls math. cpp . .. myterminal>mkdir newdir myterminal>ls math. cpp . newdir .. myterminal>cd newdir /home/ubuntu/Spring2021/CS101/Lectu re9/newdir myterminal>ls . .. myterminal>exit

Answers: 2
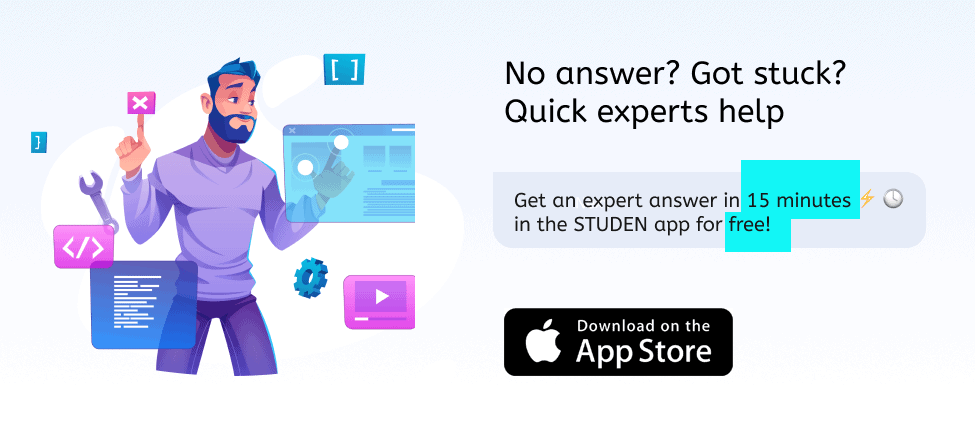

Another question on Computers and Technology

Computers and Technology, 22.06.2019 01:00
How can a broadcast station be received through cable and satellite systems?
Answers: 1

Computers and Technology, 23.06.2019 09:30
Write an essay on online collaboration, how to do it, the challenges, resolving the challenges, and consider whether the risks are greater than rewards. ( need )
Answers: 1

Computers and Technology, 24.06.2019 02:20
The first time a user launches the powerpoint program, which view is shown allowing the user to access recent presentations or create new presentations based on templates?
Answers: 1

Computers and Technology, 24.06.2019 16:00
What is a dashed line showing where a worksheet will be divided between pages when it prints? a freeze pane a split box a page break a print title
Answers: 1
You know the right answer?
A terminal program allows you to communicate with the operating system through a series of commands....
Questions

Mathematics, 28.01.2020 17:56


English, 28.01.2020 17:56


English, 28.01.2020 17:56

Computers and Technology, 28.01.2020 17:56




Mathematics, 28.01.2020 17:56

Mathematics, 28.01.2020 17:56

History, 28.01.2020 17:56




Biology, 28.01.2020 17:56

English, 28.01.2020 17:56

Mathematics, 28.01.2020 17:56

Geography, 28.01.2020 17:56