
Computers and Technology, 22.04.2021 22:20 bethzabezarahi
Write a Java application that includes the following:
Sophie, Sally and Jack's financing for their real estate development business is going well. The next step is to create a prototype Subdivision class.
Write a class Subdivision
A Subdivision has only one instance variable, an ArrayList of House elements
Subdivision has a single, no argument constructor.
Accessors
House get(int position) returns the House occupying the given position in the Subdivision. The first house added to the Subdivision is position 0, the next house added is position 1, etc.
ArrayList < House > list() returns the list of houses in this subdivision. The houses in the returned list are in the same order as they were added to the subdivision.
ArrayList < House > listByArea(double floor, double ceiling) returns the list of houses whose total area is greater than or equal to floor, and less than or equal to ceiling. The houses in the returned list are in the same order as they were added to the subdivision. If there are no such houses in the subdivision, an empty list is returned.
ArrayList < House > listByBedrooms(int floor, int ceiling) returns the list of houses that have greater than or equal to floor bedrooms, and less than or equal to ceiling bedrooms. The houses in the returned list are in the same order as they were added to the subdivision. If there are no such houses in the subdivision, an empty list is returned.
int size() returns the number of houses in the Subdivision
String toString() returns a String representation of the Subdivision
Mutators
boolean add(House houseToAdd) adds a House to the Subdivision. add returns true if the House was added successfully, false otherwise.
Test Class
Write a class Phase1 that has only a main method. Phase1 is a console program that asks the user for the number of houses to be added to a Subdivision. For each House, the program asks the user for that House's information and adds it to the Subdivision . When all the houses have been entered, it prints the size of the Subdivision , and the contents of Subdivision ordered by position. The program asks the user for floor and ceiling values for total area, and displays the houses whose total area is between or equal to those values. It similarly asks the user for floor and ceiling values for the number of bedrooms, and displays the houses whose number of bedrooms is between the floor and ceiling (inclusive).
Grading Elements
Subdivision has a single instance variable of type ArrayList
Subdivision has a single no argument constructor
All methods return the designated type and have correct signatures
All methods return the correct values
Returned lists have the proper order of House elements
Accessors do not change the state of the Subdivision (i. e., they do not change the instance variable)
add places Houses into the Subdivision at the correct position
Phase1 provides the specified operation to test Subdivision

Answers: 2
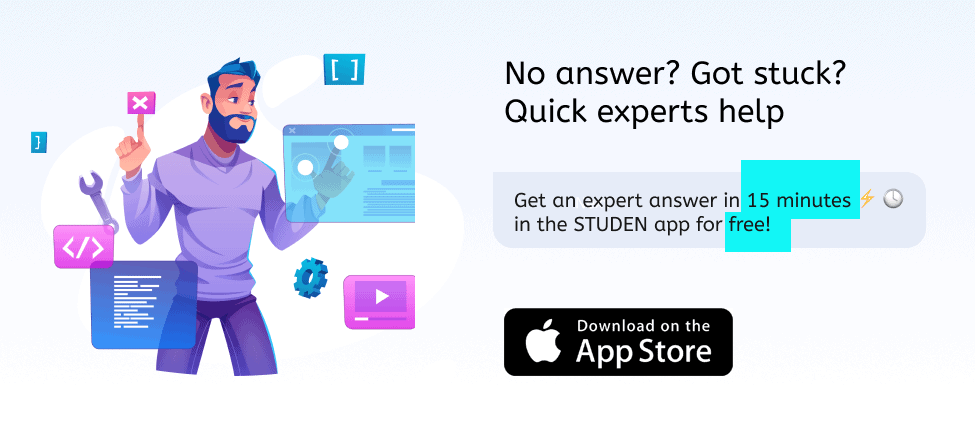

Another question on Computers and Technology

Computers and Technology, 21.06.2019 15:00
Look at the circuit illustrated in the figure above. assume that the values of r1 and r2 are equal. if you connect your meter’s test probes to points a and b in the circuit, which of the following voltages would you measure
Answers: 1

Computers and Technology, 22.06.2019 02:10
3. (5 points) describe what would be printed by the code below or what error would occur. const char* cstr = "0123456"; const char* ptr = & cstr[4]; cout < < ptr[-1] < < ptr < < endl; 1 4. (5 points) theseus has been trapped in a maze with a minotaur, which is trying to capture him. each round, theseus and the minotaur move through the maze; theseus towards the exit, and the minotaur towards theseus. theseus can move in any of the four cardinal directions, or he can wait for a round to see how the minotaur moves. write code that creates a data type to represent the possible moves that theseus could make.
Answers: 3

Computers and Technology, 23.06.2019 03:30
Ihave a singular monitor that is a tv for my computer. recently, i took apart my computer and put it back together. when i put in the hdmi cord and booted the computer to see if it worked, the computer turned on fine but the screen was blue with "hdmi no signal." i've tried everything that doesn't require buying spare parts, any answer is appreciated!
Answers: 1

Computers and Technology, 23.06.2019 14:30
The basic work area of the computer is it screen that you when you first fire up your computer
Answers: 1
You know the right answer?
Write a Java application that includes the following:
Sophie, Sally and Jack's financing for their...
Questions

Geography, 29.04.2021 04:30

Chemistry, 29.04.2021 04:30

Mathematics, 29.04.2021 04:30

Spanish, 29.04.2021 04:30

Mathematics, 29.04.2021 04:30

Biology, 29.04.2021 04:30



History, 29.04.2021 04:30


Mathematics, 29.04.2021 04:30


English, 29.04.2021 04:30

Mathematics, 29.04.2021 04:30

Mathematics, 29.04.2021 04:30



Mathematics, 29.04.2021 04:30

Mathematics, 29.04.2021 04:30