
Computers and Technology, 04.04.2021 05:40 chrisraptorofficial
SHOW ALL YOUR WORK. REMEMBER THAT PROGRAM SEGMENTS ARE TO BE WRITTEN IN JAVA.
Assume that the classes listed in the Java Quick Reference have been imported where appropriate.
Unless otherwise noted in the question, assume that parameters in method calls are not null and that methods are called only when their preconditions are satisfied.
In writing solutions for each question, you may use any of the accessible methods that are listed in classes defined in that question. Writing significant amounts of code that can be replaced by a call to one of these methods will not receive full credit.
The following class represents a customer. The variable name represents the name of the customer, and the variable currAccNum represents the customer’s account number. Each time a Customer object is created, the static variable nextAccNum is used to assign the customer’s account number.
public class Customer
{
private static int nextAccNum = 1;
private String name;
private int currAccNum;
public Customer(String n)
{
name = n;
currAccNum = nextAccNum;
nextAccNum++;
}
}
(a) Write a method for the Customer class that that will return a string representing a bill notice when passed a double value representing an amount due.
For example, if the customer has name "Jeremiah", has account number 3, and has amount due 50.50, the method should return a string in the following format.
Jeremiah, account number 3, please pay $50.50
Write the method below. Your implementation must conform to the example above.
(b) Write a method for the Customer class that returns the value of the next account number that will be assigned.
Write the method below.
(c) A student has written the following method to be included in the Customer class. The method is intended to update the name of a customer but does not work as intended.
public void updateName(String name)
{
name = name;
}
Write a correct implementation of the updateName method that avoids the error in the student’s implementation.
Write the method below.

Answers: 1
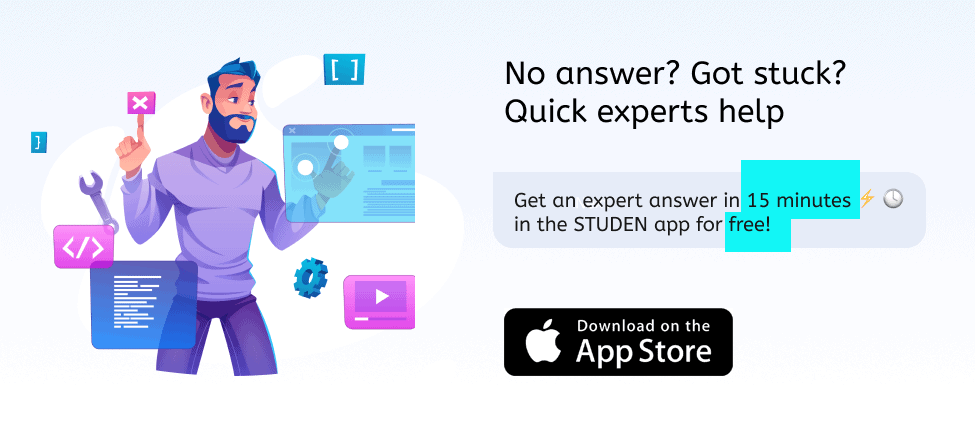

Another question on Computers and Technology

Computers and Technology, 22.06.2019 11:00
The editing of digital photos us about the same level of difficulty as editing an analog photo
Answers: 2

Computers and Technology, 22.06.2019 15:30
Why would a programmer use the logical operator and in an if statement? a: when an action is to be taken that requires both conditions to be falseb: when an action is to be taken that requires both conditions to be truec: when an action is to be taken that requires the first condition to be falsed: when an action is to be taken that requires the second condition to be truei took the test and the answer is b.
Answers: 3

Computers and Technology, 22.06.2019 23:00
Which factor is the most important when choosing a website host? whether customers will make secure transactions the number of email accounts provided the purpose of the website the quality of the host control panel
Answers: 3

Computers and Technology, 23.06.2019 03:10
Fill in the following program so that it will correctly calculate the price of the orange juice the user is buying based on the buy one get one sale.#include //main functionint main() { int cartons; float price, total; //prompt user for input information printf("what is the cost of one container of oj in dollars? \n"); scanf(" [ select ] ["%d", "%c", "%f", "%lf"] ", & price); printf("how many containers are you buying? \n"); scanf(" [ select ] ["%d", "%c", "%f", "%lf"] ", & cartons); if ( [ select ] ["cartons / 2", "cartons % 1", "cartons % 2", "cartons % price", "cartons / price", "cartons / total"] [ select ] ["=", "==", "! =", "< =", "> =", "< "] 0) total = [ select ] ["price * cartons", "cartons * price / 2 + price", "(cartons / 2) * price", "cartons / (2.0 * price)", "(cartons / 2.0) * price + price", "((cartons / 2) * price) + price"] ; else total = ((cartons / 2) * price) + price; printf("the total cost is $%.2f.\n", total); return 0; }
Answers: 2
You know the right answer?
SHOW ALL YOUR WORK. REMEMBER THAT PROGRAM SEGMENTS ARE TO BE WRITTEN IN JAVA.
Assume that the class...
Questions

Mathematics, 12.01.2021 20:10




Chemistry, 12.01.2021 20:10

Mathematics, 12.01.2021 20:10


Mathematics, 12.01.2021 20:10



English, 12.01.2021 20:10


Mathematics, 12.01.2021 20:10

English, 12.01.2021 20:10




Mathematics, 12.01.2021 20:10
