
Computers and Technology, 27.03.2021 01:20 xxblablaz799
This project is adapted from Programming project 5 on Page 520 of the textbook. We practice working on dynamic arrays to solve this problem.
You run four computer labs. Each lab contains certain number of computer stations to be determined at runtime.
Your program should keep track of the status of each computer station, whether it's empty or used by some user. Whenever a user (identified by 5-digit ID number) logs in or logs off, the program updates its data, and displays the new status of the lab.
In addition to the login and logoff, your program also supports a search command that looks up whether a certain user is using any computer station or not.
Your program then needs to dynamically allocate space for the current labs array of arrays using the entry in labsizes that corresponds (same index) to the labs[index] for the size. Please start with the starter code as it controls the Menu and showLabs displays.
Please implement the following functions:
void createArrays(IntPtr labs[], int labsizes[]); // should dynamically allocate the arrays.
void freeArrays(IntPtr labs[]); // should free the arrays
void search(IntPtr labs[], int labsizes[]); // should search for the user in the lab
void logout(IntPtr labs[], int labsizes[]); // should logout the user from the lab
Don't forget to add a comment at the top of the function to explain what it does.
Example output
An example execution of the program is displayed below:
Welcome to the LabMonitorProgram!
Please enter the number of computer stations in each lab:
How many computers in Lab 1?4
How many computers in Lab 2?5
How many computers in Lab 3?6
How many computers in Lab 4?4
MAIN MENU
0) Quit
1) Simulate login
2) Simulate logout
3) Search
1
Enter the 5 digit ID number of the user logging in:
33333
Enter the lab number the user is logging in from (1-4):
3
Enter computer station number the user is logging in to (1-6):
3
LAB STATUS
Lab # Computer Stations
1 1: empty 2: empty 3: empty 4: empty
2 1: empty 2: empty 3: empty 4: empty 5: empty
3 1: empty 2: empty 3: 33333 4: empty 5: empty 6: empty
4 1: empty 2: empty 3: empty
MAIN MENU
0) Quit
1) Simulate login
2) Simulate logout
3) Search
1
Enter the 5 digit ID number of the user logging in:
22222
Enter the lab number the user is logging in from (1-4):
2
Enter computer station number the user is logging in to (1-5):
2
LAB STATUS
Lab # Computer Stations
1 1: empty 2: empty 3: empty 4: empty
2 1: empty 2: 22222 3: empty 4: empty 5: empty
3 1: empty 2: empty 3: 33333 4: empty 5: empty 6: empty
4 1: empty 2: empty 3: empty
MAIN MENU
0) Quit
1) Simulate login
2) Simulate logout
3) Search
3
Enter the 5 digit ID number of the user logging in:
22222
User 22222 logged in Lab 2 at computer 2
MAIN MENU
0) Quit
1) Simulate login
2) Simulate logout
3) Search
2
Enter the 5 digit ID number of the user logging in:
11111
User not logged in.
LAB STATUS
Lab # Computer Stations
1 1: empty 2: empty 3: empty 4: empty
2 1: empty 2: 22222 3: empty 4: empty 5: empty
3 1: empty 2: empty 3: 33333 4: empty 5: empty 6: empty
4 1: empty 2: empty 3: empty 4: empty
MAIN MENU
0) Quit
1) Simulate login
2) Simulate logout
3) Search
2
Enter the 5 digit ID number of the user logging in:
33333
Logout user 33333 in Lab 3 at computer 3
LAB STATUS
Lab # Computer Stations
1 1: empty 2: empty 3: empty 4: empty
2 1: empty 2: 22222 3: empty 4: empty 5: empty
3 1: empty 2: empty 3: empty 4: empty 5: empty 6: empty
4 1: empty 2: empty 3: empty 4: empty
MAIN MENU
0) Quit
1) Simulate login
2) Simulate logout
3) Search
0
Bye!
Hints
You should work on your program so that it's always compilable: i. e, work on one function at a time, implement it, test it and modify the function until it works. Only then, you move on to next one.
Write comments to 1) document your algorithms and design, 2) make your code reabable, 3) debug code.
Started code link https://onlinegdb. com/Ad6Hu4jgS . it was too long thats why i could not paste it

Answers: 1
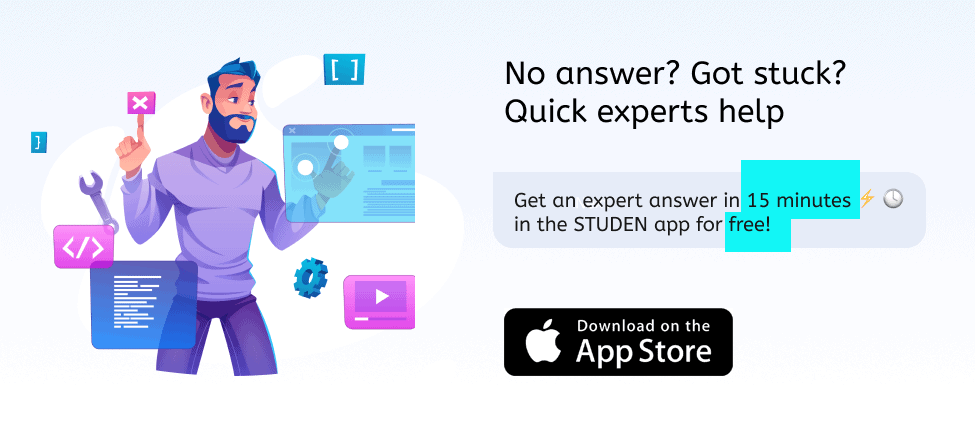

Another question on Computers and Technology

Computers and Technology, 22.06.2019 10:20
Shown below is the start of a coding region within the fist exon of a gene. 5'--3' 3'--5' how many cas9 pam sequences are present?
Answers: 1

Computers and Technology, 23.06.2019 06:30
Which option correctly describes a dbms application? a. software used to manage databases b. software used to organize files and folders c. software used to develop specialized images d. software used to create effective presentations
Answers: 1

Computers and Technology, 24.06.2019 20:00
Avirus enters a computer or network as code embedded in other software directly from another computer
Answers: 1

Computers and Technology, 24.06.2019 22:10
Function name: poly parameters: int returns: int description: a polynomial of degree n with coefficients a0,a1,a2,a3, . . ,an is the function p(x) = a0+a1x+a2x2+a3 ∗ x3+ . . +an ∗ xn this function can be evaluated at different values of x. for example, if: p(x) = 1+2x+ x2, then p(2) = 1+2 ∗ 2+22 = 9. if p(x) = 1+x2+x4, then p(2) = 21 and p(3) = 91. write a function poly() that takes as input a list of coefficients a0, a1, a2, a3, . . , an of a polynomial p(x) and a value x. the function will return poly(x), which is the value of the polynomial when evaluated at x.
Answers: 3
You know the right answer?
This project is adapted from Programming project 5 on Page 520 of the textbook. We practice working...
Questions


Mathematics, 11.05.2021 17:40



Geography, 11.05.2021 17:40


Mathematics, 11.05.2021 17:40

Mathematics, 11.05.2021 17:40





Chemistry, 11.05.2021 17:40


Computers and Technology, 11.05.2021 17:40


Mathematics, 11.05.2021 17:40

Mathematics, 11.05.2021 17:40

Social Studies, 11.05.2021 17:40