
Computers and Technology, 19.03.2021 18:20 dreamdancekay
Design a stack class by importing the available java. util. Stack to have the following features:
push(x) -- push element x onto stack, where x is anywhere between Integer. MIN_VALUE and Integer. MAX_VALUE.
pop() -- remove the element on top of the stack.
top() -- get the top element.
getMax() -- retrieve the max element in the stack in constant time (i. e., O(1)).
Your code should have the following shape and form, all in one .java file. Note the styling and documentation API already included for the target class, MaxStack:
import java. util. Stack;
public class HomeworkAssignment1_1 {
public static void main(String[] args) {
// Your main() is not graded so you can
// have any implementation in this area
MaxStack obj = new MaxStack();
obj. push(12);
obj. push(1);
obj. push(-12);
obj. pop();
System. out. println(obj. top());
System. out. println(obj. getMax());
// etc.
}
}
/**
* The MaxStack program implements a Stack class with the following features:
* push(x) -- push element x onto stack
* pop() -- remove the element on top of the stack
* top() -- get the top element.
* getMax() -- retrieve the max element in the stack in constant time (i. e., O(1)
*/
class MaxStack {
// Initialize your data structure in constructor
// or here; choice is yours.
public MaxStack() { // YOUR CODE HERE }
public void push(int x) { // YOUR CODE HERE }
public void pop() { // YOUR CODE HERE }
public int top() { // YOUR CODE HERE }
public int getMax() { // YOUR CODE HERE }
}
EXAMPLES
MaxStack maxStack = new MaxStack();
maxStack. push(-2);
maxStack. push(0);
maxStack. push(-3);
maxStack. getMax(); // returns 0
maxStack. pop();
maxStack. top(); // returns 0
maxStack. getMax(); // returns 0
CONSTRAINTS AND ASSUMPTIONS
For this problem you are ONLY allowed to use Java's reference class Stack (Links to an external site.). Failure to do so will receive 5 points off.
MaxStack does not mean elements have to be ordered in increasing or decreasing values in the Stack.
HINTS
You solution should persist a global max value while maintaining the ability to transact on a java. util. Stack instance wrapped in your MaxStack class.
When implementing the MaxStack methods, you will have to invoke your Java stack instance's comparable methods (naming convention may be different).

Answers: 2
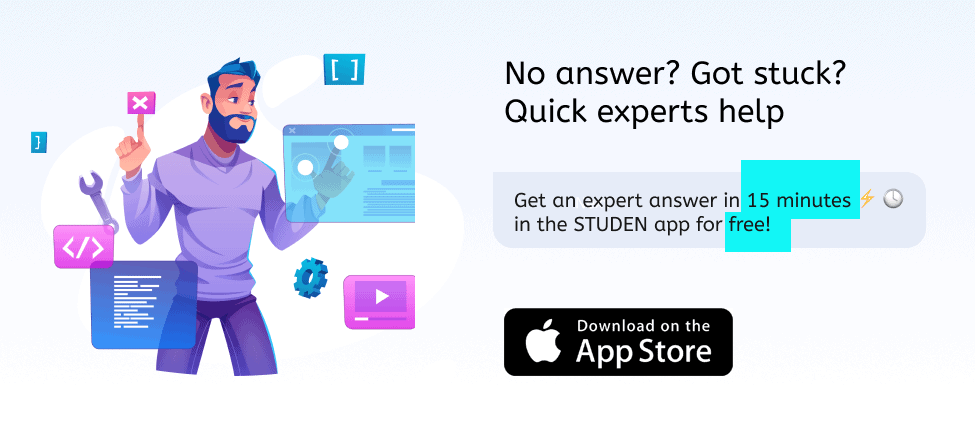

Another question on Computers and Technology

Computers and Technology, 22.06.2019 08:00
Someone with this coz i don’t really know what i can choose, just pick whatever u want. homework - you need to choose a website that you like or use frequently. you must visit the website and discuss 6 different features/parts/aspects of the website that you think makes it good. (100 words)
Answers: 2

Computers and Technology, 24.06.2019 07:00
Why would a business likely use a java applet - to back up their data files for the business - to create a program that a customer can launch in their web browser - to create music on a powerpoint presentation - to organize files on their company directory
Answers: 3

Computers and Technology, 24.06.2019 08:30
Formatting rows and columns is similar to cell formatting. in an openoffice calc spreadsheet, you can format data entered into rows and columns with the of the rows and columns options. you can insert rows and columns into, or delete rows and columns from, a spreadsheet. use the insert or delete rows and columns option on the insert tab. alternatively, select the row or column where you want new rows or columns to appear, right-click, and select insert only row or only column options. you can hide or show rows and columns in a spreadsheet. use the hide or show option on the format tab. for example, to hide a row, first select the row, then choose the insert tab, then select the row option, and then select hide. alternatively, you can select the row or columns, right-click, and select the hide or show option. you can adjust the height of rows and width of columns. select row and then select the height option on the format tab. similarly, select column, then select the width option on the format tab. alternatively, you can hold the mouse on the row and column divider, and drag the double arrow to the position. you can also use the autofit option on the table tab to resize rows and columns.
Answers: 1

Computers and Technology, 24.06.2019 10:00
3. what do the terms multipotentialite, polymath, or scanner mean?
Answers: 2
You know the right answer?
Design a stack class by importing the available java. util. Stack to have the following features:
p...
Questions

Mathematics, 19.05.2021 02:20



Mathematics, 19.05.2021 02:20



Business, 19.05.2021 02:20






Mathematics, 19.05.2021 02:20


Mathematics, 19.05.2021 02:20

Mathematics, 19.05.2021 02:20

Mathematics, 19.05.2021 02:20


Mathematics, 19.05.2021 02:20

History, 19.05.2021 02:20