Infix to Postfix
Write a C++ program that will accept infix expressions (like 5*(4+8)) and
co...

Computers and Technology, 25.02.2021 19:00 minasotpen1253
Infix to Postfix
Write a C++ program that will accept infix expressions (like 5*(4+8)) and
convert them to postfix. You are to use Dijkstra's algorithm for converting.
Dijkstra's Algorithm:
Read in 1 line into a string
Print the string
while there are characters left to process in the string
| get a token (skip over blanks)
| if the token is a digit then output(token)
| else
|
| | if the token is '(' then push(token)
| | else
| |
| | | if the token is ')' then
| | |
| | | | while the top item is not '('
| | | | pop(temp) and output(temp);
| | | | pop(temp)
| | |
| | | else
| | |
| | | | if the stack is empty then push(token)
| | | | else
| | | |
| | | | | while the stack is not empty
| | | | | and the priority (token) <= priority (top item on the stack)
| | | | | pop(temp) and output(temp)
| | | | | push(token)
| | | |
| | |
| |
|
while the stack is not empty do pop(temp) and output(temp)
Precedence of the Operators:
operators : ^ * / + - (
precedence: 3 2 2 1 1 0
where ^ means exponentiation
input for the assignment:
2 + 3 * 5
2 + 3 * 5 ^ 6
2 + 3 - 5 + 6 - 4 + 2 - 1
2 + 3 * (5 - 6) - 4
2 * 3 ^ 5 * 6 - 4
(2 + 3) * 6 ^ 2
Output for the assignment
1: 2 + 3 * 5
235*+
2: 2 + 3 * 5 ^ 6
2356^*+
3: 2 + 3 - 5 + 6 - 4 + 2 - 1
23+5-6+4-2+1-
4: 2 + 3 * (5 - 6) - 4
2356-*+4-
5: 2 * 3 ^ 5 * 6 - 4
235^*6*4-
6: (2 + 3) * 6 ^ 2
23+62^*
You might also try:
7: ( ( ( ( 2 + 3 - 4 ) / 2 + 8 ) * 3 * ( 4 + 5 ) / 2 / 3 + 9 ) )
23+4-2/8+3*45+*2/3/9+
Programming Notes:
You are to write a well-composed program. The stack routines are to be
defined as methods in a class and are to be in a separate file.
//A sample c++ program to read a file 1 line at a time
#include
#include
#include
using namespace std;
int main()
{
string aline;
ifstream inData;
inData. open("infix. data");
while ( getline(inData, aline) )
{
cout << aline << endl;
}
inData. close();
}

Answers: 1
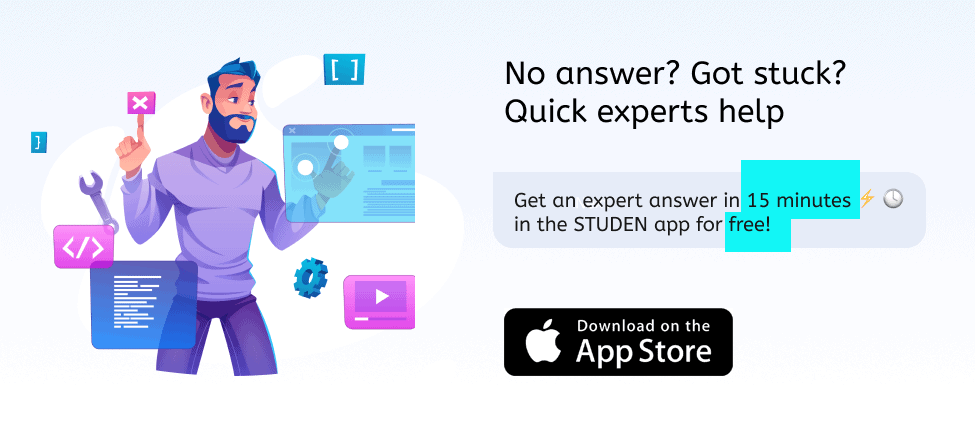

Another question on Computers and Technology

Computers and Technology, 22.06.2019 22:00
Perform the following tasks: a. create a class named testclass that holds a single private integer field and a public constructor. the only statement in the constructor is one that displays the message “constructing”. write a main()function that instantiates one object of the testclass. save the file as testclass.cpp in the chapter 08 folder. run the program and observe the results. b. write another main()function that instantiates an array of 10 testclass objects. save the file as test class array.c . run this program and observe the results.
Answers: 1

Computers and Technology, 23.06.2019 12:00
Which of these is an example of an integrated presentation? a. a table created in powerpoint b. an image pasted into powerpoint c. a caption created in powerpoint d. an excel chart pasted into powerpoint
Answers: 1

Computers and Technology, 23.06.2019 20:40
On nba 2k 19, every time i try to join a my park game, it leads ro a website telling my dad that he needs ps plus. i already have ps plus though. how do i fix this?
Answers: 2

Computers and Technology, 24.06.2019 11:00
Need fast im timed in a paragraph of 125 words, explain at least three ways that engineers explore possible solutions in their projects.
Answers: 2
You know the right answer?
Questions

Mathematics, 03.07.2019 19:00







Social Studies, 03.07.2019 19:00



Mathematics, 03.07.2019 19:00

History, 03.07.2019 19:00


Mathematics, 03.07.2019 19:00

History, 03.07.2019 19:00

Health, 03.07.2019 19:00



Social Studies, 03.07.2019 19:00

Mathematics, 03.07.2019 19:00