
Computers and Technology, 31.01.2021 22:50 MarishaTucker
Please help!
Consider this implementation of the class Card, that stores information related to a cards monetary value, name, condition, and number in a set of collectible cards:
public class Card
{
private double value;
private String name;
private int setNum; //each card has its own, unique setNum
private String condition;
public Card (String nom, String cond, double val, int numSet){
name = nom;
condition = cond;
value = val;
setNum = numSet;
}
public String getName(){
return name;
}
public String getCondition(){
return condition;
}
public double getValue(){
return value;
}
public int getSetNum(){
return setNum;
}
public String toString()
{
return name + ":: " + setNum;
}
}
A subsequent class CardCollection has been created with the instance variable ArrayList collection that stores a collection of cards.
import java. util. ArrayList;
public class CardCollection
{
ArrayList collection;
double totalVal;
public CardCollection(ArrayList myColl)
{
collection = myColl;
}
public double totalValue()
{
// code intentionally left blank
// you will write this method in #1
}
public String checkPerfect()
{
// code intentionally left blank
// you will write this method in #2
}
public ArrayList orderNumerically()
{
System. out. println("Before sort: " + collection);
// code intentionally left blank
System. out. println("After sort: " + sortedCards);
return collection;
}
}
The driver class CardRunner is shown below:
import java. util. ArrayList;
public class CardRunner
{
public static void main (String[] args)
{
ArrayList x = new ArrayList ();
// you will write this code in #3
x. add(new Card("Downey", "new", 48.72, 7));
x. add(new Card("Jones", "perfect", 200, 5));
x. add(new Card("Smith", "perfect", 24.8, 3));
x. add(new Card("Peek", "good", 13.5, 1));
System. out. println("\nThe total value of my collection is: $" + // #4 );
System. out. println("\nMy perfect cards are: " + // code intentionally left blank);
System. out. println("\n" + // code intentionally left blank);
}
}
1) Write a method totalValue() for CardCollection which returns the total value of all cards in a card collection.
2) Write a method checkPerfect() for CardCollection that prints the name of the Cards in a collection that are in "perfect" condition. 3) Instantiate a CardCollection object which could be used to access methods from the CardCollection class.
4) Complete this programming statement from CardRunner
System. out. println("\nThe total value of my collection is: $" + // #4 );

Answers: 1
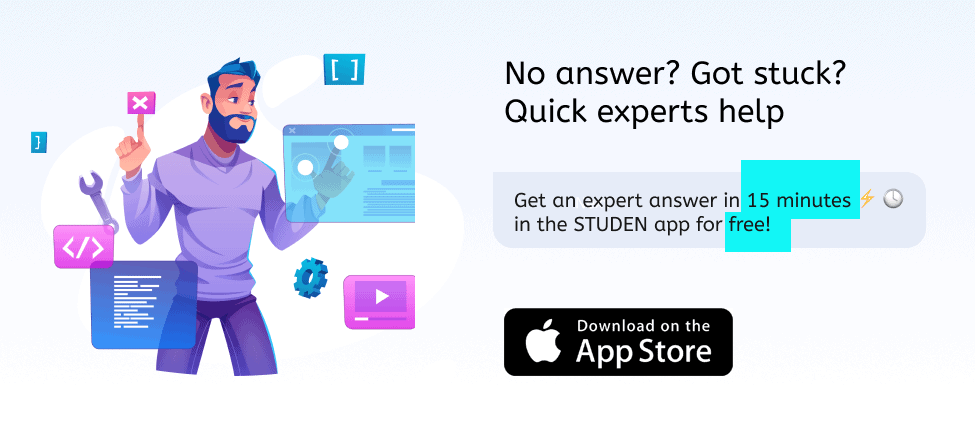

Another question on Computers and Technology

Computers and Technology, 22.06.2019 21:30
Write a function named printfloatrepresentation(float number) that will print the floating point representation of a number using the format given below. (sign bit) exponent in binary (assumed bit).significandfor example if the number passed an argument is 71 yourprogram should print (0) 10000101 (1).00011100000000000000000similarly if the number passed to the function as argument is -71 the program should print (1) 10000101 (1).00011100000000000000000
Answers: 3

Computers and Technology, 23.06.2019 04:00
Write a method that takes in an array of point2d objects, and then analyzes the dataset to find points that are close together. be sure to review the point2d api. in your method, if the distance between any pair of points is less than 10, display the distance and the (x,y)s of each point. for example, "the distance between (3,5) and (8,9) is 6.40312." the complete api for the point2d adt may be viewed at ~pf/sedgewick-wayne/algs4/documentation/point2d.html (links to an external site.)links to an external site.. try to write your program directly from the api - do not review the adt's source code.
Answers: 1

Computers and Technology, 24.06.2019 13:30
Consider jasper’s balance sheet. which shows how to calculate jasper’s net worth?
Answers: 1

Computers and Technology, 24.06.2019 18:30
Jacking is a crime that takes place when a hacker misdirects url to a different site. the link itself looks safe, but the user is directed to an unsafe page
Answers: 1
You know the right answer?
Please help!
Consider this implementation of the class Card, that stores information related to a c...
Questions



Mathematics, 03.08.2019 07:30

Computers and Technology, 03.08.2019 07:30




English, 03.08.2019 07:30



Chemistry, 03.08.2019 07:30


Mathematics, 03.08.2019 07:30





History, 03.08.2019 07:30
