
Computers and Technology, 27.12.2020 16:50 kelbruggie
Introduction
For this project, you will implement an RPN calculator that reads a series of expressions in RPN form from a file. The user has two options: they can either enter the filename as a command line argument or they may be prompted for a filename after launching the program. In either case, the program halts if the file does not exist.
Individual expressions in RPN format (see below) are read from the file. If an expression is well-formed the program will evaluate it and display the expression along with its results on the console. Malformed expressions result in an error message being displayed on the console.
Overview
Infix notation is the notation commonly used in arithmetical and logical formulae and statements. It is characterized by the placement of operators between operandsâ"infixed operators"âsuch as the plus sign in 2 + 2. Infix notation is more difficult to parse by computers than prefix notation (e. g. + 2 2) or postfix notation (e. g. 2 2 +). However many programming languages use it due to its familiarity.
In infix notation, unlike in prefix or postfix notations, parentheses surrounding groups of operands and operators are necessary to indicate the intended order in which operations are to be performed. In the absence of parentheses, certain precedence rules determine the order of operations.
Reverse Polish Notation (RPN), also known as postfix notation, is a mathematical notation in which operators follow their operands, in contrast to Polish Notation (PN), in which operators precede their operands. Expressions in this form do not require any parentheses as long as each operator has a fixed number of operands.
In postfix notation, the operators follow their operands. For example, to add 3 and 4, you would write 3 4 + rather than 3 + 4. If there are multiple operations, operators are provided immediately after their second operands. The expression written 3 â 4 + 5 in conventional notation would be written 3 4 â 5 + in RPN: 4 is first subtracted from 3, then 5 is added to it. A distinct advantage of RPN is that it removes the need for parentheses that are required by infix notation.
RPN Algorithm
for each token in the postfix expression:
if token is an operator:
operand_2 â pop from the stack
operand_1 â pop from the stack
result â evaluate token with operand_1 and operand_2
push result back onto the stack
else if token is an operand:
push token onto the stack
end_for
result â pop from the stack
Program Requirements
Design a program with the following specifications:
Required Program Name: RPN. java
The program can use either command line arguments or standard input (the CLI) to enter in a file name. For standard input, the program should use standard output to prompt the user for the name of a file. This file contains a set of expressions in RPN format.
If the input file does not exist or an I/O error occurs while processing it, an error message is displayed and execution halts.
The input data file is a plain ASCII text file containing RPN expressions of varying length and complexity.
Each expression is evaluated and any malformed expressions generate an error message.
Program output should be neat and adequately spaced for readability.
Program Implementation
Using a Stack ADT, implement the program as a Java application within a single compilation unit.
Using only integer values, implement the following operators: addition (+), subtraction (-), multiplication (*), integer division(/) and positive number exponentiation (^).
For internal documentation, use plenty of comments throughout the program.
Be sure to include your name and course/section in the application documentation block.
For grading, submit a copy of the source code and a screen snapshot of the program execution.

Answers: 2
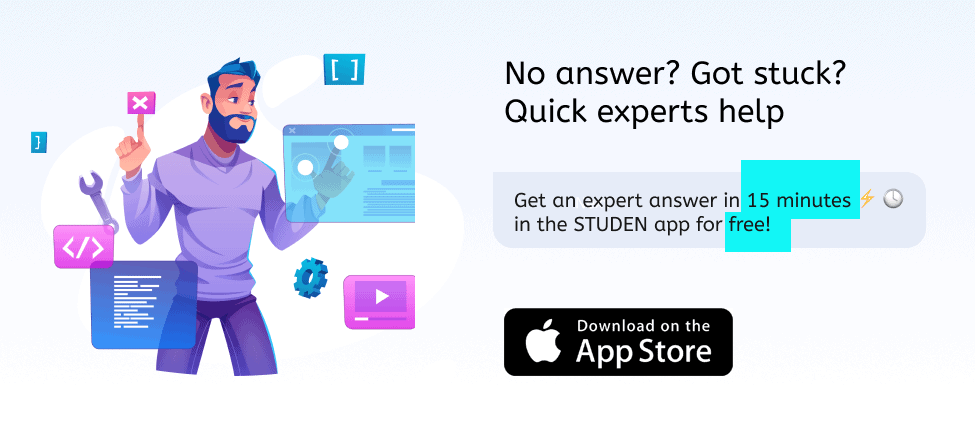

Another question on Computers and Technology

Computers and Technology, 23.06.2019 00:30
Which one of the following is the most accurate definition of technology? a electronic tools that improve functionality b electronic tools that provide entertainment or practical value c any type of tool that serves a practical function d any type of tool that enhances communication
Answers: 1

Computers and Technology, 23.06.2019 06:00
When is a chart legend used a. all the time b. whenever you are comparing data that is the same c. whenever you are comparing multiple sets of data d. only for hand-drawn charts
Answers: 2

Computers and Technology, 24.06.2019 03:30
Explain the importance of html in web page designing in 20 sentences..
Answers: 1

Computers and Technology, 24.06.2019 05:00
Who is most likely be your target audience if you create a slide presentation that had yellow background and purple text
Answers: 2
You know the right answer?
Introduction
For this project, you will implement an RPN calculator that reads a series of expressi...
Questions

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30


Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30



Geography, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Social Studies, 09.12.2021 09:30


History, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30

Mathematics, 09.12.2021 09:30