
Computers and Technology, 09.11.2020 16:40 meiyrarodriguez
String Basics. Write this assignment in C.
YOU CAN'T USE ANY BUILT-IN STRING FUNCTIONS.
Write a function that takes strSource as a parameter. The function will return the length of the string.
Write a subroutine that takes strDestination and strSource as parameters. The subroutine will copy the source string to the destination string.
Write a function that takes strSource and chrLetterToFind as parameters. The function will return the index of the first occurrence of the letter (case sensitive) in the string searching from left to right. Return –1 if the letter is not in the string.
Write a function that takes strSource and chrLetterToFind as parameters. The function will return the index of the first occurrence of the letter (case INsensitive) in the string searching from left to right. Return –1 if the letter is not in the string. Do NOT change the source string.
Write a subroutine that takes strDestination and strSource as parameters. The subroutine will append the source string to the end of the destination string. Assume the destination string is large enough to hold all the characters.
Write a subroutine that takes strDestination and strSource as parameters. The subroutine will copy the source string to the destination string in reverse order. Do NOT change the source string.
Write a subroutine that takes strDestination and strSource as parameters. The subroutine will copy the source string to the destination string and make the destination string all uppercase. Do NOT change the source string.
Write a subroutine that takes strDestination, strSource, intStartIndex and intLength as parameters. The subroutine will copy a substring from the source string to the destination string starting at intStartIndex and of length intLength. Do NOT change the source string.
Write a function that takes strSource as a parameter. The function will return the number of words in the string. For example the sentence "Mary had a little lamb." has 5 words. Counting words is not the same thing as counting spaces. Do NOT change the source string.
Call each of the above functions from your main subroutine and display the results. For example:
void main( )
{
char strSource[ 50 ] = "I Love Star Trek";
int intLength = 0;
char strDestination[ 50 ] = "";
// Problem #1: String length
intLength = StringLength( "I Love Star Trek" );
printf( "Problem #1: String Length: %d\n", intLength );
printf( "\n" );
// Problem #2: CopyString
CopyString( strDestination, "I Love Star Trek" );
printf( "Problem #2: CopyString: %s\n", strDestination );
printf( "\n" );
etc
}

Answers: 2
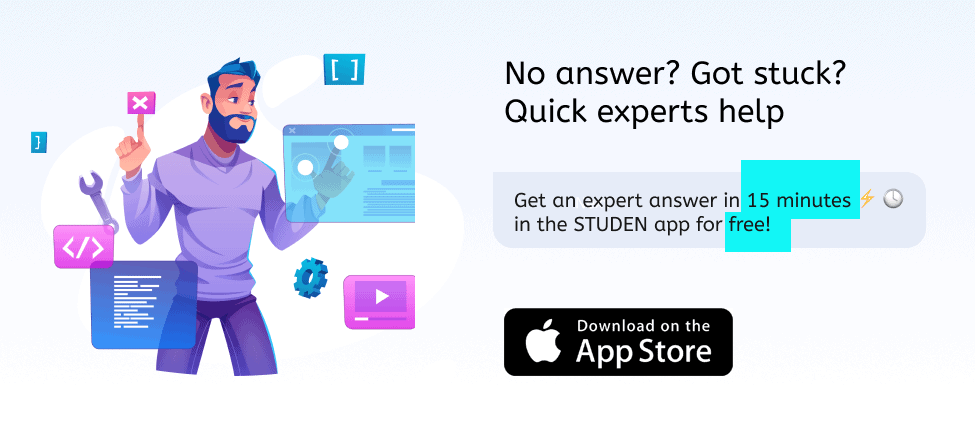

Another question on Computers and Technology

Computers and Technology, 22.06.2019 20:00
Which type of file can be used to import data into a spreadsheet?
Answers: 1

Computers and Technology, 23.06.2019 11:00
How should you specify box sizes on a web page if you want the boxes to vary according to the font size of the text they contain? a. in pixels b. in inches c. as percentages d. in em units
Answers: 2

Computers and Technology, 23.06.2019 12:00
What does the level 1 topic in a word outline become in powerpoint? a. first-level bullet item b. slide title c. third-level bullet item d. second-level bullet item
Answers: 1

Computers and Technology, 23.06.2019 22:20
Learning sign language is an example of a(n) learning sign language is an example of a(n)
Answers: 2
You know the right answer?
String Basics. Write this assignment in C.
YOU CAN'T USE ANY BUILT-IN STRING FUNCTIONS.
Questions




Health, 24.08.2019 23:30


Computers and Technology, 24.08.2019 23:30


Mathematics, 24.08.2019 23:30

Biology, 24.08.2019 23:30

Social Studies, 24.08.2019 23:30

Physics, 24.08.2019 23:30

Biology, 24.08.2019 23:30

Social Studies, 24.08.2019 23:30

Mathematics, 24.08.2019 23:30

Mathematics, 24.08.2019 23:30



