
Computers and Technology, 24.10.2020 05:20 talanna394
7.27 LAB: Artwork label (classes/constructors)
Given main(), complete the Artist class (in files Artist. h and Artist. cpp) with constructors to initialize an artist's information, get member functions, and a PrintInfo() member function. The default constructor should initialize the artist's name to "None" and the years of birth and death to 0. PrintInfo() should display Artist Name, born if the year of death is -1 or Artist Name (-) otherwise.
Complete the Artwork class (in files Artwork. h and Artwork. cpp) with constructors to initialize an artwork's information, get member functions, and a PrintInfo() member function. The constructor should by default initialize the title to "None", the year created to 0. Declare a private field of type Artist in the Artwork class.
Ex. If the input is:
Pablo Picasso
1881
1973
Three Musicians
1921
the output is:
Artist: Pablo Picasso (1881-1973)
Title: Three Musicians, 1921
If the input is:
Brice Marden
1938
-1
Distant Muses
2000
the output is:
Artist: Brice Marden, born 1938
Title: Distant Muses, 2000
READ ONLY main. cpp
#include "Artist. h"
#include "Artwork. h"
#include
#include
using namespace std;
int main(int argc, const char* argv[]) {
string userTitle, userArtistName;
int yearCreated, userBirthYear, userDeathYear;
getline(cin, userArtistName);
cin >> userBirthYear;
cin. ignore();
cin >> userDeathYear;
cin. ignore();
getline(cin, userTitle);
cin >> yearCreated;
cin. ignore();
Artist userArtist = Artist(userArtistName, userBirthYear, userDeathYear);
Artwork newArtwork = Artwork(userTitle, yearCreated, userArtist);
newArtwork. PrintInfo();
}
Artist. h
#ifndef ARTISTH
#define ARTISTH
#include
using namespace std;
class Artist{
public:
Artist();
Artist(string artistName, int birthYear, int deathYear);
string GetName() const;
int GetBirthYear() const;
int GetDeathYear() const;
void PrintInfo() const;
private:
// TODO: Declare private data members - artistName, birthYear, deathYear
};
#endif
Artist. cpp
#include "Artist. h"
#include
#include
using namespace std;
// TODO: Define default constructor
// TODO: Define second constructor to initialize
// private fields (artistName, birthYear, deathYear)
// TODO: Define get functions: GetName(), GetBirthYear(), GetDeathYear()
// TODO: Define PrintInfo() function
// If deathYear is entered as -1, only print birthYear
Artwork. h
#ifndef ARTWORKH
#define ARTWORKH
#include "Artist. h"
#include
using namespace std;
class Artwork{
public:
Artwork();
Artwork(string title, int yearCreated, Artist artist);
string GetTitle();
int GetYearCreated();
void PrintInfo();
private:
// TODO: Declare private data members - title, yearCreated
// TODO: Declare private data member artist of type Artist
};
#endif
Artwork. cpp
#include "Artist. h"
// TODO: Define default constructor
// TODO: Define second constructor to initialize
// private fields (title, yearCreated, artist)
// TODO: Define get functions: GetTitle(), GetYearCreated()
// TODO: Define PrintInfo() function

Answers: 1
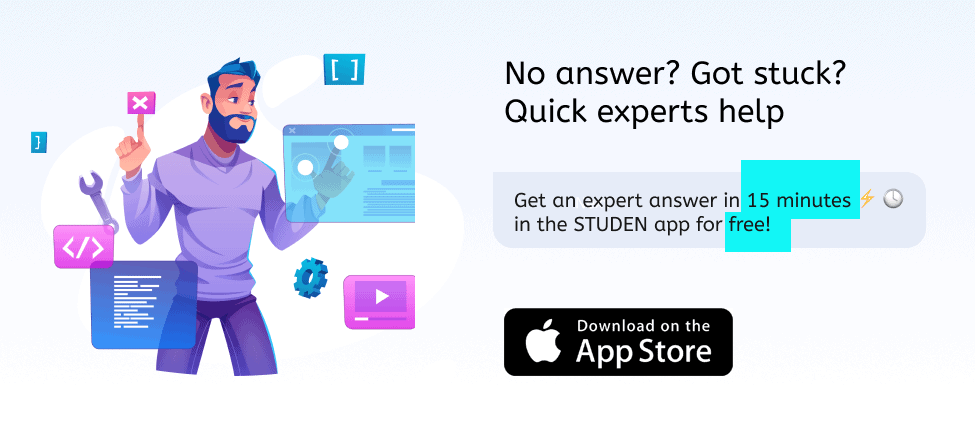

Another question on Computers and Technology

Computers and Technology, 23.06.2019 13:30
Spoons are designed to be used for: spring hammering. applying body filler. identifying high and low spots. sanding highly formed areas.
Answers: 3

Computers and Technology, 23.06.2019 15:20
What does a bonus object do? a. subtracts lives b. keeps track of a player's health c. gives a player an advantage d. makes text appear
Answers: 1

Computers and Technology, 24.06.2019 01:30
Could you find out how im still getting an 83 percent on this in edhesive a = input("enter an animal: ") s = input ("enter a sound: ") e = "e-i-e-i-o" print ("old macdonald had a farm, " + e) print ("and on his farm he had a " + a + "," + e) print ("with a " + s + "-" + s + " here and a " + s + "-" + s + " there") print ("here a " + s+ " there a " + s) print ("everywhere a " + s + "-" + s ) print ("old macdonald had a farm, " + e)
Answers: 2

Computers and Technology, 24.06.2019 16:00
What is a dashed line showing where a worksheet will be divided between pages when it prints? a freeze pane a split box a page break a print title
Answers: 1
You know the right answer?
7.27 LAB: Artwork label (classes/constructors)
Given main(), complete the Artist class (in files Ar...
Questions

Mathematics, 18.12.2020 18:40




Mathematics, 18.12.2020 18:40





Mathematics, 18.12.2020 18:40



English, 18.12.2020 18:40


History, 18.12.2020 18:40

Mathematics, 18.12.2020 18:40

English, 18.12.2020 18:40

Geography, 18.12.2020 18:40

Mathematics, 18.12.2020 18:40

Arts, 18.12.2020 18:40