
Computers and Technology, 20.10.2020 21:01 tylermorse3775
1. Write a program that determines if the user can ride a rollercoaster.
To ride the rollercoaster, you must be at least 42 inches tall. You must also be at least 9 years old.
The code is already written to ask the user how tall and old they are.
Write the code to determine if they can ride the rollercoaster.
If they can, print âWelcome aboard!â
If they cannot, print âSorry, you are not eligible to rideâ
import java. util. Scanner;
public class RideTheRollerCoaster
{
public static void main(String[] args)
{
Scanner input = new Scanner(System. in);
System. out. println("How tall in inches are you? " );
int height = input. nextInt();
System. out. println("How old are you? " );
int age = input. nextInt();
//Start your code here.
}
}
2. Write a program that asks the user for three strings.
Then, print out whether or not the first string concatenated to the second string is equal to the third string. Donât worry about spaces at the beginning or ending of strings unless you want to! The code for the user to input the three strings is already written for you.
Here are a few sample program runs:
Sample Program 1:
First string? pepper
Second string? mint
Third string? peppermint
pepper + mint is equal to peppermint!
Sample Program 2:
First string? go
Second string? fish
Third string? donuts
go + fish is not equal to donuts!
import java. util. Scanner;
public class ThreeStrings
{
public static void main(String[] args)
{
Scanner input = new Scanner(System. in);
System. out. println("First String? ");
String first = input. nextLine();
System. out. println("Second String? ");
String second = input. nextLine();
System. out. println("Third String? ");
String third = input. nextLine();
//Start your code here.
}
}

Answers: 3
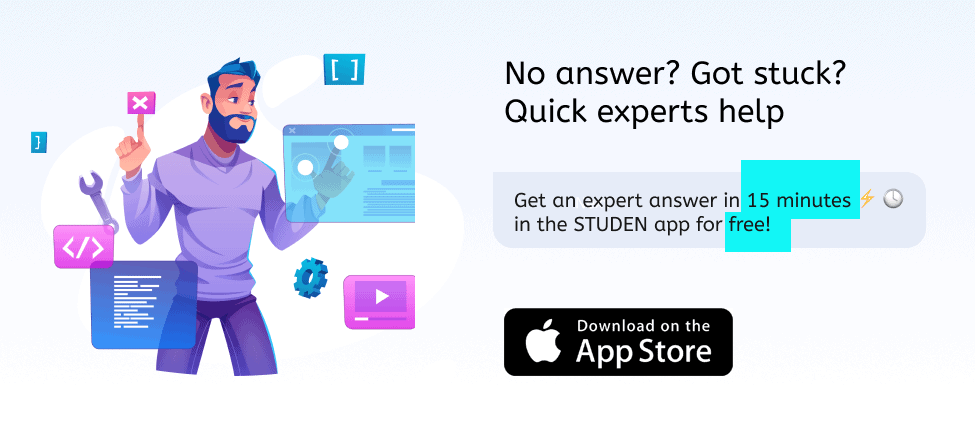

Another question on Computers and Technology

Computers and Technology, 22.06.2019 05:00
Which two editions of windows 7 support 64 bit cpus? choose two out of professional, business, starter, or home premium.
Answers: 1

Computers and Technology, 22.06.2019 18:00
What is the first view you place in your drawing?
Answers: 1

Computers and Technology, 22.06.2019 22:50
Which is the best minecraft server? a. mineplex b. worldonecraft c. 9b9t d. 2b2t
Answers: 2

You know the right answer?
1. Write a program that determines if the user can ride a rollercoaster.
To ride the rollercoaster,...
Questions

Chemistry, 02.06.2021 22:30


Biology, 02.06.2021 22:30


Mathematics, 02.06.2021 22:30

Mathematics, 02.06.2021 22:30

Mathematics, 02.06.2021 22:30

Mathematics, 02.06.2021 22:30



Computers and Technology, 02.06.2021 22:30




Mathematics, 02.06.2021 22:30

Mathematics, 02.06.2021 22:30

Mathematics, 02.06.2021 22:30

Computers and Technology, 02.06.2021 22:30

Mathematics, 02.06.2021 22:30