
Computers and Technology, 16.10.2020 05:01 dude3328
#include
using namespace std;
const int SIZE = 4;
bool isSorted(const int arr[], int size);
bool isNonDecreasing(const int arr[], int size);
bool isNonIncreasing(const int arr[], int size);
void printArr(const int arr[], int size);
int main()
{
int test1[] = { 4, 7, 10, 69 };
int test2[] = { 10, 9, 7, 3 };
int test3[] = { 19, 12, 23, 7 };
int test4[] = { 5, 5, 5, 5 };
if (!isSorted(test1, SIZE))
cout << "NOT ";
cout << "SORTED" << endl;
printArr(test1, SIZE);
if (!isSorted(test2, SIZE))
cout << "NOT ";
cout << "SORTED" << endl;
printArr(test2, SIZE);
if (!isSorted(test3, SIZE))
cout << "NOT ";
cout << "SORTED" << endl;
printArr(test3, SIZE);
if (!isSorted(test4, SIZE))
cout << "NOT ";
cout << "SORTED" << endl;
printArr(test4, SIZE);
return 0;
}
bool isSorted(const int arr[], int size)
{
// TODO: This function returns true if the array is sorted. It could be
// sorted in either non-increasing (descending) or non-decreasing (ascending)
// order. If the array is not sorted, this function returns false.
// HINT: Notice that the functions isNonDecreasing and isNonIncreasing are not
// called from main. Call the isNonDecreasing and isNonIncreasing functions here.
}
bool isNonDecreasing(const int arr[], int size)
{
// TODO: Loop through the array to check whether it is sorted in
// non-decreasing (in other words, ascending) order. If the array
// is non-decreasing, return true. Otherwise, return false.
}
bool isNonIncreasing(const int arr[], int size)
{
// TODO: Loop through the array to check whether it is sorted in
// non-increasing (in other words, descending) order. If the array
// is non-increasing, return true. Otherwise, return false.
}
void printArr(const int arr[], int size)
{
for (int i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl << endl;
}

Answers: 2
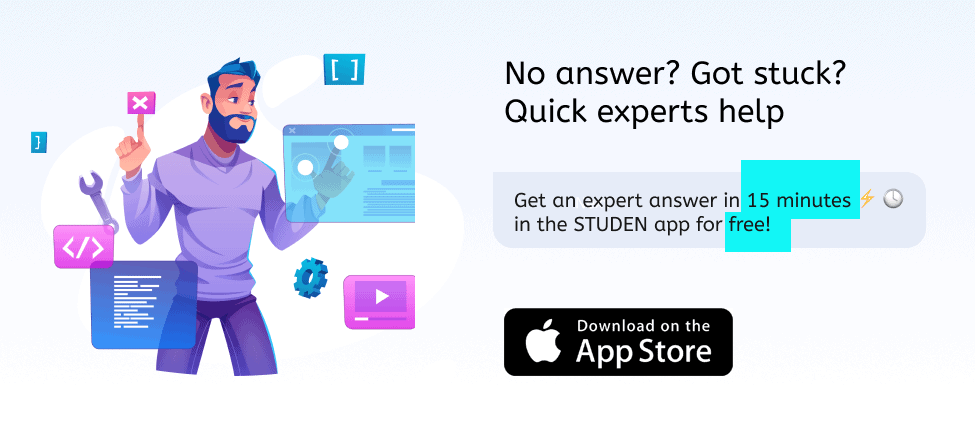

Another question on Computers and Technology

Computers and Technology, 22.06.2019 18:00
Write a method named addall that could be placed inside the hashintset class. this method accepts another hashintset as a parameter and adds all elements from that set into the current set, if they are not already present. for example, if a set s1 contains [1, 2, 3] and another set s2 contains [1, 7, 3, 9], the call of s1.addall(s2); would change s1 to store [1, 2, 3, 7, 9] in some order. you are allowed to call methods on your set and/or the other set. do not modify the set passed in. this method should run in o(n) time where n is the number of elements in the parameter set passed in.
Answers: 2

Computers and Technology, 22.06.2019 22:30
Who needs to approve a change before it is initiated? (select two.) -change board -client or end user -ceo -personnel manager -project manager
Answers: 1

Computers and Technology, 23.06.2019 09:00
Before you record your own voice, you should a. record other people's voices b. warm up and practice difficult names c. listen to your favorite songs d. read a transcript of a good radio news segment
Answers: 1

Computers and Technology, 23.06.2019 09:30
Light travels at a speed of 186,000 miles a second. the distance light travels in a year is 5,865,690,000,000 miles/year 5,865,695,000,000 miles/year 58,656,950,000,000 miles/year 6,789,000,0000 miles/year
Answers: 1
You know the right answer?
#include
using namespace std;
const int SIZE = 4;
bool isSorted(const int arr[], int si...
const int SIZE = 4;
bool isSorted(const int arr[], int si...
Questions

Mathematics, 18.10.2020 08:01

English, 18.10.2020 08:01


Geography, 18.10.2020 08:01


Mathematics, 18.10.2020 08:01


Mathematics, 18.10.2020 08:01


Mathematics, 18.10.2020 08:01

Physics, 18.10.2020 08:01

Mathematics, 18.10.2020 08:01


Mathematics, 18.10.2020 08:01


Biology, 18.10.2020 08:01

Mathematics, 18.10.2020 08:01



Mathematics, 18.10.2020 08:01