
Computers and Technology, 23.09.2020 16:01 wilboisa000
Write a program to read a list of exam grades given as int's in the range of 0 to 100. Your program will display the total number of grades and the number of grades in each letter-grade category as follows:
A93 <= grade <= 100 A- 90 <= grade < 93 B+ 87 <= grade < 90 B 83 <= grade < 87 B- 80 <= grade < 83 C+ 77 <= grade < 80 C 73 <= grade < 77 C- 70 <= grade < 73 D 60 <= grade < 70 F 0 <= grade < 60 Use a negative number as a sentinel value to indicate the end of the input. (The negative value is used only to end the loop, do not use it in your calculations.) Each time you prompt the user to enter a grade you will print: Enter a grade:
For example, if the input is: 98 95 87 86 83 92 85 78 74 72 81 71 69 63 50 43 -1 The output would be:
Total number of grades = 16
Number of A's = 2
Number of A-'s = 1
Number of B+'s = 1
Number of B's = 3
Number of B-'s = 1
Number of C+'s = 1
Number of C's = 1
Number of C-'s = 2
Number of D's = 2
Number of F's = 2
The highest score is 98
The lowest score is 50
The average is 75.5
This is what I have so far and it is not working correctly:
public static void main(String[] args) {
// scanner
Scanner scnr =new Scanner (System. in);
//ints grades and count
int x;
int A = 0;
int B = 0;
int C = 0;
int D = 0;
int F = 0;
int count = 1;
//int min max total
int min, max;
int total = 0 ;
//double
double average;
//prompt user for input
System. out. print("Please enter the exam scores as integer ");
System. out. print("percentages in the rage 0-100. ");
System. out. println("Please end the list with a negative integer.");
//scnr
x = scnr. nextInt();
min = x;
max = x;
//while loop
while (x >= 0){
x = scnr. nextInt();
if (x >= 0){
total = total + x;
count++;
if (x < min)
min = x;
if (x > min)
max = x; }
while (x >= 90 && x <= 100) {
x = scnr. nextInt();
A++;
//Grade B
if (x >= 80 && x <= 89)
B++;
//Grade C
if (x >= 70 && x <= 79)
C++;
//Grade D
if (x >= 60 && x <= 69)
D++;
//Grade F
if (x >= 0 && x <= 59)
F++;
}
}
// average
average = total/count;
//results/output
System. out. println("Total number of grades: " + count);
System. out. println("Number of A's: " + A);
System. out. println("Number of B's: " + B);
System. out. println("Number of C's: " + C);
System. out. println("Number of D's: " + D);
System. out. println("Number of F's: " + F);
System. out. println("Highest score: " + max);
System. out. println("Lowest score: " + min);
System. out. println("Average: " + average);
}
}

Answers: 3
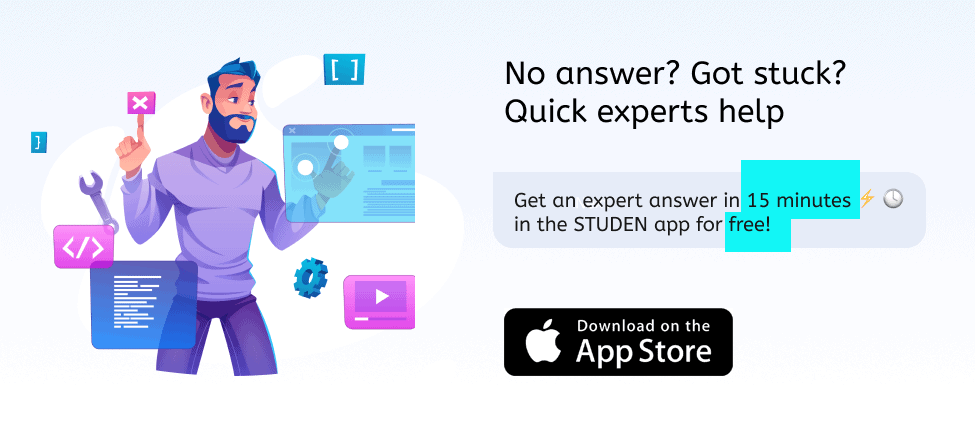

Another question on Computers and Technology

Computers and Technology, 23.06.2019 04:31
Selling a product through an electronic medium is
Answers: 1

Computers and Technology, 23.06.2019 15:00
In the blank libreoffice writer document, to start the process of entering a date field into a letter, click on the insert menu. edit menu. file menu. fields menu.
Answers: 3

Computers and Technology, 23.06.2019 19:00
Acompany is hiring professionals for web designing. the firm is small with few resources. they want employees who possess problem-solving skills and can independently carry out responsibilities. which kind of employee should they select?
Answers: 2

Computers and Technology, 23.06.2019 22:50
An environmental protection agency study of 12 automobiles revealed a correlation of 0.47 between engine size and emissions. at 0.01 significance level, can we conclude that there is a positive association between the variables? what is the p value? interpret.
Answers: 2
You know the right answer?
Write a program to read a list of exam grades given as int's in the range of 0 to 100. Your program...
Questions


History, 04.08.2019 19:00

Mathematics, 04.08.2019 19:00

English, 04.08.2019 19:00

History, 04.08.2019 19:00




History, 04.08.2019 19:00

History, 04.08.2019 19:00

Mathematics, 04.08.2019 19:00

Mathematics, 04.08.2019 19:00



Mathematics, 04.08.2019 19:00

Social Studies, 04.08.2019 19:00

Chemistry, 04.08.2019 19:00

Biology, 04.08.2019 19:00

Biology, 04.08.2019 19:00

Biology, 04.08.2019 19:00