
Computers and Technology, 23.09.2020 05:01 jasontbyrer
The base class Pet has private fields petName, and petAge. The derived class Dog extends the Pet class and includes a private field for dogBreed. Complete main() to:
create a generic pet and print information using printInfo().
create a Dog pet, use printInfo() to print information, and add a statement to print the dog's breed using the getBreed() method.
Ex. If the input is:
Dobby
2
Kreacher
3
German Schnauzer
The output is:
Pet Information:
Name: Dobby
Age: 2
Pet Information:
Name: Kreacher
Age: 3
Breed: German Schnauzer
(File is marked as read-only)
Dog. java
public class Dog extends Pet {
private String dogBreed;
public void setBreed(String userBreed) {
dogBreed = userBreed;
}
public String getBreed() {
return dogBreed;
}
}
(File is marked as read-only)
Pet. java
public class Pet {
protected String petName;
protected int petAge;
public void setName(String userName) {
petName = userName;
}
public String getName() {
return petName;
}
public void setAge(int userAge) {
petAge = userAge;
}
public int getAge() {
return petAge;
}
public void printInfo() {
System. out. println("Pet Information: ");
System. out. println(" Name: " + petName);
System. out. println(" Age: " + petAge);
}
}
PetInformation. java
import java. util. Scanner;
public class PetInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System. in);
Pet myPet = new Pet();
Dog myDog = new Dog();
String petName, dogName, dogBreed;
int petAge, dogAge;
petName = scnr. nextLine();
petAge = scnr. nextInt();
scnr. nextLine();
dogName = scnr. next();
dogAge = scnr. nextInt();
scnr. nextLine();
dogBreed = scnr. nextLine();
// TODO: Create generic pet (using petName, petAge) and then call printInfo
myPet. setPetName(petName);
myPet. setPetAge(petAge);
myPet. printInfo();
// TODO: Create dog pet (using dogName, dogAge, dogBreed) and then call printInfo
myDog. setPetName(dogName);
myDog. setPetAge(dogAge);
myDog. setDogBreed(dogBreed);
myDog. printInfo();
// TODO: Use getBreed(), to output the breed of the dog
System. out. println(" Breed: " + myDog. getDogBreed());
}
}

Answers: 1
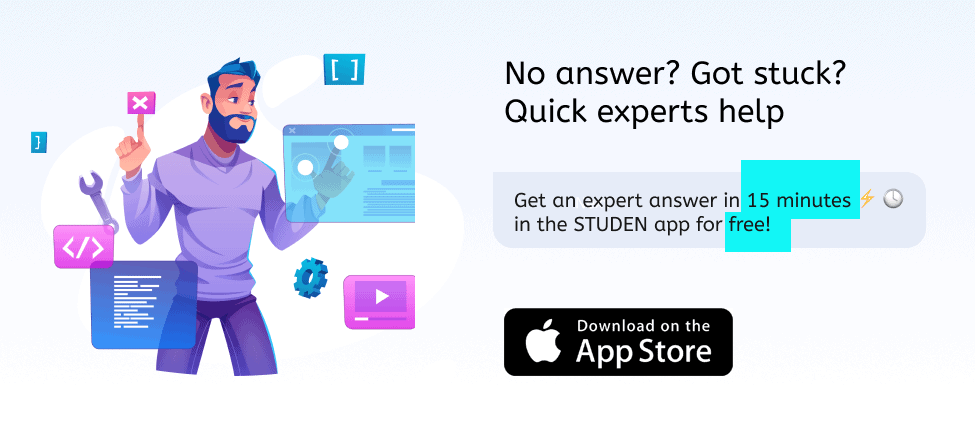

Another question on Computers and Technology

Computers and Technology, 22.06.2019 14:30
Create a pseudocode design to prompt a student for their student id and the titles of the three classes they want to add. the solution should display the student’s id and a total bill. • bill a student using the following rules: o students can only add up to 3 classes at a time.
Answers: 3

Computers and Technology, 22.06.2019 16:00
If a client wants to make minor edits, what should he/she use?
Answers: 3

Computers and Technology, 22.06.2019 18:30
Which of these options are the correct sequence of actions for content to be copied and pasted? select content, click the copy button, click the paste button, and move the insertion point to where the content needs to be inserted. click the copy button, select the content, move the insertion point to where the content needs to be inserted, and click the paste button. select the content, click the copy button, move the insertion point to where the content needs to be inserted, and click the paste button. select the content, move the insertion point to where the content needs to be inserted, click the copy button, and click the paste button.
Answers: 3

Computers and Technology, 23.06.2019 08:00
The managing director of a company sends a christmas greeting to all his employees through the company email. which type of network does he use? he uses an .
Answers: 3
You know the right answer?
The base class Pet has private fields petName, and petAge. The derived class Dog extends the Pet cla...
Questions







Mathematics, 10.05.2020 04:57

Mathematics, 10.05.2020 04:57


English, 10.05.2020 04:57



Mathematics, 10.05.2020 04:57

Mathematics, 10.05.2020 04:57




