
Computers and Technology, 19.09.2020 01:01 astra
Java programming.
Implement the Shape hierarchy shown in Fig. 9.3 of the textbook. Each TwoDimensionalShape should contain method getArea to calculate the area of the two-dimensional shape. Each ThreeDimensionalShape should have methods getArea and getVolume to calculate the surface area and volume, respectively, of the three-dimensional shape. Do not implement a class for Tetrahedron
Create a program that uses an array of Shape references to objects of each concrete class in the hierarchy. The program should print a text description of the object to which each array element refers. Also, in the loop that processes all the shapes in the array, determine whether each shape is a TwoDimensionalShape or a ThreeDimensionalShape. If a shape is a TwoDimensionalShape, display its area. If a shape is a ThreeDimensionalShape, display its area and volume.
Hints:
• Create an abstract (base) class, called Shape, as follows:
public abstract class Shape
{
private int x; // x coordinate
private int y; // y coordinate
// two-argument constructor
public Shape( int x, int y )
{
this. x = x;
this. y = y;
} // end two-argument Shape constructor
// set x coordinate
public void setX( int x )
{
this. x = x;
} // end method setX
// set y coordinate
public void setY( int y )
{
this. y = y;
} // end method setY
// get x coordinate
public int getX()
{
return x;
} // end method getX
// get y coordinate
public int getY()
{
return y;
} // end method getY
// return String representation of Shape object
public String toString()
{
return String. format( "(%d, %d)", getX(), getY() );
}
// abstract methods
public abstract String getName();
} // end class Shape
The constructors of the subclasses will have the following arguments:
public abstract class TwoDimensionalShape extends Shape
{
private int dimension1;
private int dimension2;
public TwoDimensionalShape( int x, int y, int d1, int d2 )
{
super( x, y );
dimension1 = d1;
dimension2 = d2;
} // end four-argument TwoDimensionalShape constructor
…
public class Circle extends TwoDimensionalShape
{
// three-argument constructor
public Circle( int x, int y, int radius )
{
super( x, y, radius, radius );
} // end three-argument Circle constructor
……
public class Square extends TwoDimensionalShape
{
// three-argument constructor
public Square( int x, int y, int side )
{
super( x, y, side, side );
} // end three-argument Square constructor
……
public abstract class ThreeDimensionalShape extends Shape
{
private int dimension1;
private int dimension2;
private int dimension3;
// five-argument constructor
public ThreeDimensionalShape(int x, int y, int d1, int d2, int d3 )
{
super( x, y );
dimension1 = d1;
dimension2 = d2;
dimension3 = d3;
} // end five-argument ThreeDimensionalShape constructor
……
public class Sphere extends ThreeDimensionalShape
{
// three-argument constructor
public Sphere( int x, int y, int radius )
{
super( x, y, radius, radius, radius );
} // end three-argument Shape constructor
……
public class Cube extends ThreeDimensionalShape
{
// three-argument constructor
public Cube( int x, int y, int side )
{
super( x, y, side, side, side );
} // end three-argument Cube constructor
……
ï‚· Use the following class as a driver class
public class ShapeTest
{
// create Shape objects and display their information
public static void main( String args[] )
{
Shape shapes[] = new Shape[ 4 ];
shapes[ 0 ] = new Circle( 22, 88, 4 );
shapes[ 1 ] = new Square( 71, 96, 10 );
shapes[ 2 ] = new Sphere( 8, 89, 2 );
shapes[ 3 ] = new Cube( 79, 61, 8 );
// call method print on all shapes
for ( Shape currentShape : shapes )
{
System. out. printf( "%s: %s",currentShape. getName(), currentShape );
if ( currentShape instanceof TwoDimensionalShape )
{
TwoDimensionalShape twoDimensionalShape =
( TwoDimensionalShape ) currentShape;
System. out. printf( "%s's area is %s\n",
currentShape. getName(), twoDimensionalShape. getArea() );
} // end if
if ( currentShape instanceof ThreeDimensionalShape )
{
ThreeDimensionalShape threeDimensionalShape =
( ThreeDimensionalShape) currentShape;
System. out. printf( "%s's area is %s\n",
currentShape. getName(), threeDimensionalShape. getArea() );
System. out. printf( "%s's volume is %s\n",
currentShape. getName(),
threeDimensionalShape. getVolume() );
}
System. out. println();
} // end for
} // end main
} // end class ShapeTest

Answers: 3
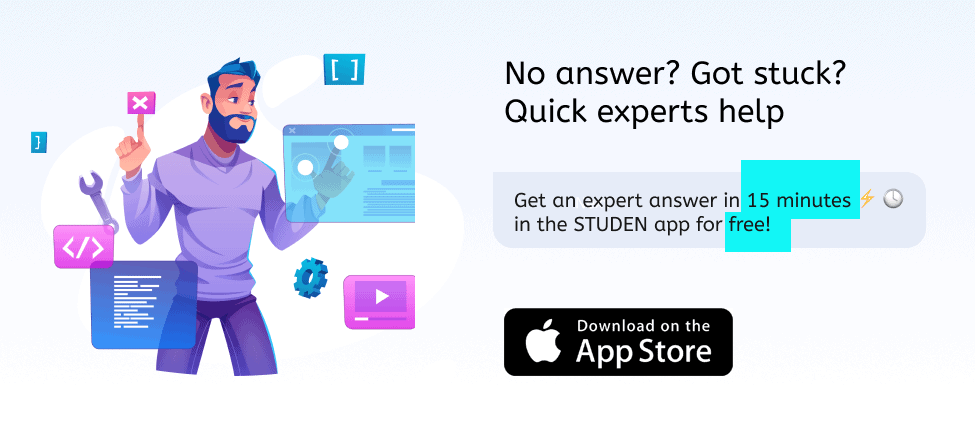

Another question on Computers and Technology

Computers and Technology, 23.06.2019 01:30
For a typical middle-income family, what is the estimated cost of raising a child to the age of 18? $145,500 $245,340 $304,340 $455,500
Answers: 2

Computers and Technology, 23.06.2019 06:30
On early television stations, what typically filled the screen from around 11pm until 6am? test dummies test patterns tests testing colors
Answers: 1

Computers and Technology, 23.06.2019 16:00
An english teacher would like to divide 8 boys and 10 girls into groups, each with the same combination of boys and girls and nobody left out. what is the greatest number of groups that can be formed?
Answers: 2

Computers and Technology, 24.06.2019 13:00
Why should you evaluate trends when thinking about a career path?
Answers: 1
You know the right answer?
Java programming.
Implement the Shape hierarchy shown in Fig. 9.3 of the textbook. Each TwoDimensio...
Questions






Mathematics, 11.05.2021 01:00

Biology, 11.05.2021 01:00


English, 11.05.2021 01:00

History, 11.05.2021 01:00


Mathematics, 11.05.2021 01:00

History, 11.05.2021 01:00





