
Computers and Technology, 13.08.2020 05:01 Thejollyhellhound20
I REALLY NEED HELP WITH SCHEME:
In Scheme, source code is data. Every non-atomic expression is written as a Scheme list, so we can write procedures that manipulate other programs just as we write procedures that manipulate lists.
Rewriting programs can be useful: we can write an interpreter that only handles a small core of the language, and then write a procedure that converts other special forms into the core language before a program is passed to the interpreter.
For example, the let special form is equivalent to a call expression that begins with a lambda expression. Both create a new frame extending the current environment and evaluate a body within that new environment. Feel free to revisit Problem 15 as a refresher on how the let form works.
(let ((a 1) (b 2)) (+ a b))
;; Is equivalent to:
((lambda (a b) (+ a b)) 1 2)
These expressions can be represented by the following diagrams:
Let Lambda
let lambda
Use this rule to implement a procedure called let-to-lambda that rewrites all let special forms into lambda expressions. If we quote a let expression and pass it into this procedure, an equivalent lambda expression should be returned: pass it into this procedure:
scm> (let-to-lambda '(let ((a 1) (b 2)) (+ a b)))
((lambda (a b) (+ a b)) 1 2)
scm> (let-to-lambda '(let ((a 1)) (let ((b a)) b)))
((lambda (a) ((lambda (b) b) a)) 1)
In order to handle all programs, let-to-lambda must be aware of Scheme syntax. Since Scheme expressions are recursively nested, let-to-lambda must also be recursive. In fact, the structure of let-to-lambda is somewhat similar to that of scheme_eval--but in Scheme! As a reminder, atoms include numbers, booleans, nil, and symbols. You do not need to consider code that contains quasiquotation for this problem.
(define (let-to-lambda expr)
(cond ((atom? expr) )
((quoted? expr) )
((lambda? expr) )
((define? expr) )
((let? expr) )
(else )))
CODE:
; Returns a function that checks if an expression is the special form FORM
(define (check-special form)
(lambda (expr) (equal? form (car expr
(define lambda? (check-special 'lambda))
(define define? (check-special 'define))
(define quoted? (check-special 'quote))
(define let? (check-special 'let))
;; Converts all let special forms in EXPR into equivalent forms using lambda
(define (let-to-lambda expr)
(cond ((atom? expr)
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
)
((quoted? expr)
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
)
((or (lambda? expr)
(define? expr))
(let ((form (car expr))
(params (cadr expr))
(body (cddr expr)))
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
))
((let? expr)
(let ((values (cadr expr))
(body (cddr expr)))
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
))
(else
; BEGIN PROBLEM 19
'replace-this-line
; END PROBLEM 19
)))

Answers: 2
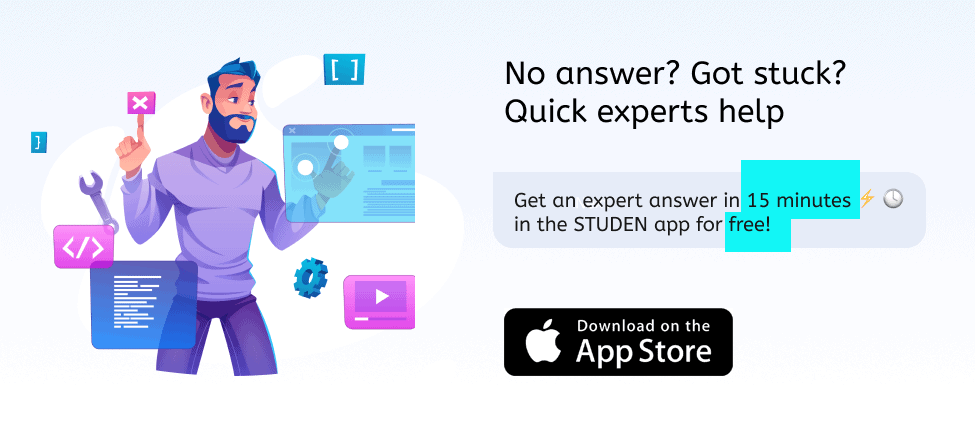

Another question on Computers and Technology

Computers and Technology, 22.06.2019 05:00
Which two editions of windows 7 support 64 bit cpus? choose two out of professional, business, starter, or home premium.
Answers: 1

Computers and Technology, 22.06.2019 18:30
All of the following are characteristics that must be contained in any knowledge representation scheme except
Answers: 3

Computers and Technology, 23.06.2019 15:00
Based on the current economic situation do you expect the employment demand for graduating engineers to increase or decrease? explain the basis for your answer. with a significant economic recovery, what do you think will happen to future enrollments in graduating engineering programs?
Answers: 1

Computers and Technology, 23.06.2019 17:00
1. which of the following is not an example of an objective question? a. multiple choice. b. essay. c. true/false. d. matching 2. why is it important to recognize the key word in the essay question? a. it will provide the answer to the essay. b. it will show you a friend's answer. c. it will provide you time to look for the answer. d. it will guide you on which kind of answer is required.
Answers: 1
You know the right answer?
I REALLY NEED HELP WITH SCHEME:
In Scheme, source code is data. Every non-atomic expression is writ...
Questions

Arts, 06.10.2020 04:01

Health, 06.10.2020 04:01



Mathematics, 06.10.2020 04:01


Chemistry, 06.10.2020 04:01

Biology, 06.10.2020 04:01


Mathematics, 06.10.2020 04:01

Mathematics, 06.10.2020 04:01



Arts, 06.10.2020 04:01

History, 06.10.2020 04:01





History, 06.10.2020 04:01