You are given a skeleton sequential program for matrix multiplication below:
#include
#inclu...

Computers and Technology, 29.07.2020 06:01 babyissy2016
You are given a skeleton sequential program for matrix multiplication below:
#include
#include
#include
#define M 500
#define N 500
int main(int argc, char *argv) {
//set number of threads here
omp_set_num_threads(8);
int i, j, k;
double sum;
double **A, **B, **C;
A = malloc(M*sizeof(double *));
B = malloc(M*sizeof(double *));
C = malloc(M*sizeof(double *));
for (i = 0; i < M; i++) {
A[i] = malloc(N*sizeof(double));
B[i] = malloc(N*sizeof(double));
C[i] = malloc(N*sizeof(double));
}
double start, end;
for (i = 0; i < M; i++) {
for (j = 0; j < N; j++) {
A[i][j] = j*1;
B[i][j] = i*j+2;
C[i][j] = j-i*2;
}
}
start = omp_get_wtime();
for (i = 0; i < M; i++) {
for (j = 0; j < N; j++) {
sum = 0;
for (k=0; k < M; k++) {
sum += A[i][k]*B[k][j];
}
C[i][j] = sum;
}
}
end = omp_get_wtime();
printf("Time of computation: %f\n", end-start);
}
You are to parallelize this algorithm in three different ways:
Add the necessary pragma to parallelize the outer for loop
Remove the pragma for the outer for loop and create a pragma for the middle for loop
3. Add the necessary pragmas to parallelize both the outer and middle for loops
and collect timing data given 2 thread, 4 threads, 8 threads, 16 threads, 32 threads and three matrix sizes. You
will find that when you run the same program several times, the timing values can vary significantly. Therefore
for each set of conditions, collect five data values and average them. Use a spreadsheet program either from MS Office or OpenOffice to collect and store your data and perform the necessary calculations and illustrate the results visually with Charts.

Answers: 2
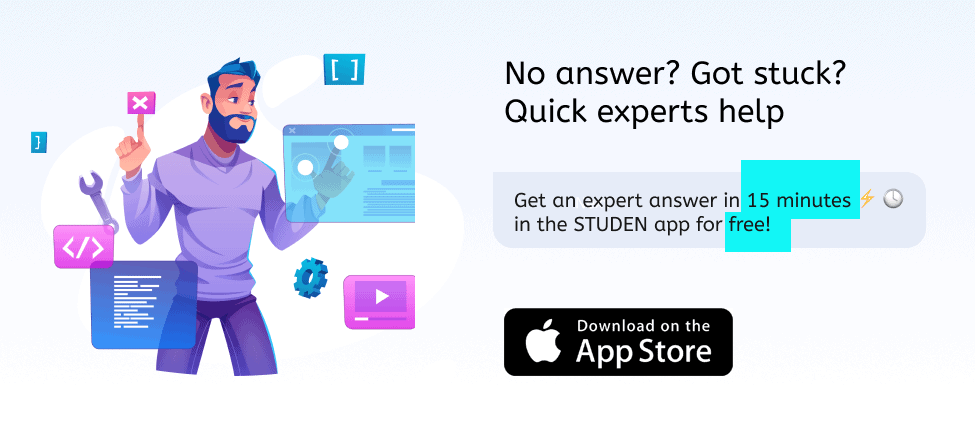

Another question on Computers and Technology

Computers and Technology, 21.06.2019 20:40
Peripherals are part of the main computer. true false
Answers: 3

Computers and Technology, 23.06.2019 02:50
There’s only one game mode that stars with the letter ‘e’ in cs: go. which of the options below is it?
Answers: 1

Computers and Technology, 23.06.2019 06:20
What is a point-in-time measurement of system performance?
Answers: 3

Computers and Technology, 23.06.2019 10:50
Your friend kayla is starting her own business and asks you whether she should set it up as a p2p network or as a client-server network. list three questions you might ask to kayla decide which network to use and how her answers to those questions would affect your recommendation.
Answers: 2
You know the right answer?
Questions





Mathematics, 02.06.2021 20:00

Arts, 02.06.2021 20:00


Mathematics, 02.06.2021 20:00




English, 02.06.2021 20:00

Social Studies, 02.06.2021 20:00

Mathematics, 02.06.2021 20:00



Mathematics, 02.06.2021 20:00


Mathematics, 02.06.2021 20:00