
Computers and Technology, 28.07.2020 20:01 vannybelly83
Create an application that reads a file that contains an email list, reformats the data, and writes the cleaned list to another file.
Below are the grading criteria…
Fix formatting. The application should fix the formatting problems.
Write the File. Your application should write a file named prospects_clean. csv.
Use title case. All names should use title case (an initial capital letter with the rest lowercase).
Fix email addresses. All email addresses should be lowercase.
Trim spaces. All extra spaces at the start or end of a string should be removed.
Here is the code work I was working on. It seems that there are errors happening and I get message that file is not found when using this code…
import java. io. IOException;
import java. io. BufferedReader;
import java. io. FileReader;
import java. util. ArrayList;
public class CSVReader {
// Used to read CSV file
public static ArrayList readCSV(String fileName) throws IOException {
String line = " ";
BufferedReader br = null;
ArrayList ar = new ArrayList();
br = new BufferedReader(new FileReader(fileName));
// Reading content from CSV file
while ((line = br. readLine()) != null) {
ar. add(line);
}
// Closing Buffered Reader
br. close();
return ar;
}
}
import java. util. ArrayList;
public class Cleaner {
// Convert to title case
static String convert(String str) {
str = str. toLowerCase();
char[] array = str. toCharArray();
// Modify first element in array.
array[0] = Character. toUpperCase(array[0]);
// Return string.
return new String(array);
}
// CSV cleaner
public ArrayList CSVCleaner(ArrayList fileContent) {
ArrayList cleanerCon = new ArrayList();
String delimiter = ",";
StringBuilder newLine = null;
for (String str : fileContent) {
String[] fullLine = str. split(delimiter);
newLine = new StringBuilder();
for (int i = 0; i < fullLine. length - 1; i++) {
newLine. append(convert(fullLine[i]) + ",");
}
if (EmailValidator. isValidEmail(fullLine[fullLine. length - 1].toLowerCase())) {
newLine. append(fullLine[fullLine. length-1].toLowerCase());
} else {
newLine. append(convert(fullLine[fullLine. length - 1]));
}
cleanerCon. add(newLine. toString());
}
return cleanerCon;
}
}

Answers: 3
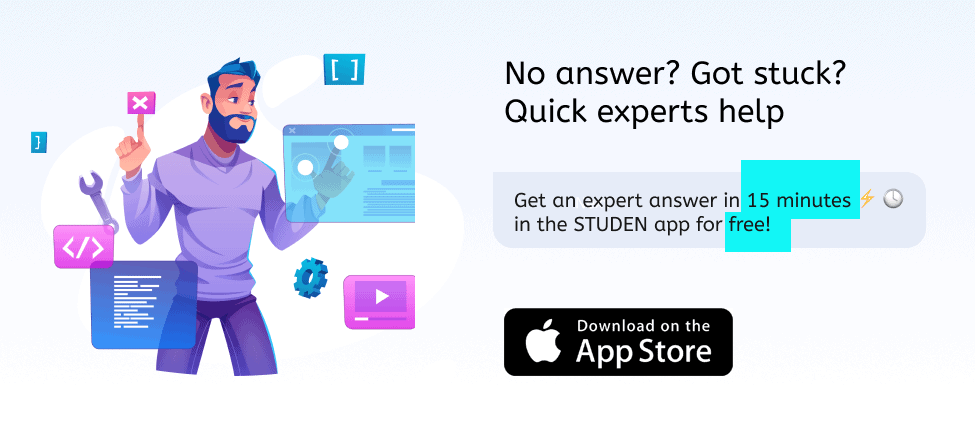

Another question on Computers and Technology

Computers and Technology, 22.06.2019 00:30
Which of the following methods could be considered a “best practice” in terms of informing respondents how their answers to an on-line survey about personal information will be protected? respondents are informed that investigators will try to keep their participation confidential; however, confidentiality cannot be assured. respondents are informed that a research assistant will transfer all the research data to a password-protected computer that is not connected to the internet, via a usb flashdrive. the computer is located in a research team member’s office. the investigator uses the informed consent process to explain her institution’s method for guaranteeing absolute confidentiality of research data. the investigator uses the informed consent process to explain how respondent data will be transmitted from the website to his encrypted database without ever recording respondents’ ip addresses, but explains that on the internet confidentiality cannot be absolutely guaranteed.
Answers: 1

Computers and Technology, 23.06.2019 13:30
Anetwork security application that prevents access between a private and trusted network and other untrusted networks
Answers: 1

Computers and Technology, 23.06.2019 15:00
In the blank libreoffice writer document, to start the process of entering a date field into a letter, click on the insert menu. edit menu. file menu. fields menu.
Answers: 3

Computers and Technology, 23.06.2019 16:00
What is the biggest difference between section breaks and regular page breaks? section breaks are more difficult to add than page breaks. section breaks make it easier for you to view the document as an outline. section breaks allow you to have areas of the document with different formatting. section breaks are smaller than regular page breaks.
Answers: 2
You know the right answer?
Create an application that reads a file that contains an email list, reformats the data, and writes...
Questions

Biology, 06.11.2020 03:40

Social Studies, 06.11.2020 03:40

Mathematics, 06.11.2020 03:40


Mathematics, 06.11.2020 03:40


Mathematics, 06.11.2020 03:40

Mathematics, 06.11.2020 03:40

Mathematics, 06.11.2020 03:40

Biology, 06.11.2020 03:40

English, 06.11.2020 03:40

Physics, 06.11.2020 03:40





Chemistry, 06.11.2020 03:40



Chemistry, 06.11.2020 03:40