
Computers and Technology, 16.04.2020 00:49 skrillex88
Concepts tested by this program Hash Table, Link List, hash code, buckets/chaining, exception handling, read/write files (FileChooser)A concordance lists every word that occurs in a document in alphabetical order, and for each word it gives the line number of every line in the document where the word occurs. Write a program that creates a concordance. There will be two ways to create a concordance. The first requires a document to be read from an input file, and the concordance data is written to an output file. The second reads the input from a string and returns an ArrayList of strings that represent the concordance of the string. Because they are so common, don't include the words "the" or "and" in your concordance. Also, do not include words that have length less than 3. Strip out all punctuation, except apostrophes that occur in the middle of a word, i. e. let’s, we’d, etc. Data Elements – ConcordanceDataElement, implements Comparable and consists of a String (the word) and a reference to a LinkedList (list of line numbers where word occurs). Follow the Javadoc provided for you. Data Structure – ConcordanceDataStructure, Implements the Interface that is provided. You will be implementing a hash table with buckets. It will be an array of linked list of ConcordanceDataElements. The add method will take a word and a line number to be added to the data structure. If the word already exists, the line number will be added to the linked list for this word. If the line number for the word already exists, don’t add it again to the linked list. (i. e. if Sarah was on line 5 twice, the first line 5 would be added to the linked list for Sarah, the second one would not). If the word doesn’t exist, create a ConcordanceDataElement and add it to the HashTable. Two constructors will be required, one that takes in an integer that is the estimated number of words in the text, the other is used for testing purposes. Look at the provided Javadoc. Data Manager – the interface that is provided. The data manager allows the client (user) to create a concordance file or a concordance list (ArrayList of strings). The input is read (from a file or string) and is added to the data structure through the add method. The add method requires a word and a line number. The line number is incremented every time a newline appears in the file or the string. Concepts tested by this program Hash Table, Link List, hash code, buckets/chaining, exception handling, read/write files (FileChooser)A concordance lists every word that occurs in a document in alphabetical order, and for each word it gives the line number of every line in the document where the word occurs. Write a program that creates a concordance. There will be two ways to create a concordance. The first requires a document to be read from an input file, and the concordance data is written to an output file. The second reads the input from a string and returns an ArrayList of strings that represent the concordance of the string. Because they are so common, don't include the words "the" or "and" in your concordance. Also, do not include words that have length less than 3. Strip out all punctuation, except apostrophes that occur in the middle of a word, i. e. let’s, we’d, etc. Data Elements – ConcordanceDataElement, implements Comparable and consists of a String (the word) and a reference to a LinkedList (list of line numbers where word occurs). Follow the Javadoc provided for you. Data Structure – ConcordanceDataStructure, Implements the Interface that is provided. You will be implementing a hash table with buckets. It will be an array of linked list of ConcordanceDataElements. The add method will take a word and a line number to be added to the data structure. If the word already exists, the line number will be added to the linked list for this word. If the line number for the word already exists, don’t add it again to the linked list. (i. e. if Sarah was on line 5 twice, the first line 5 would be added to the linked list for Sarah, the second one would not). If the word doesn’t exist, create a ConcordanceDataElement and add it to the HashTable. Two constructors will be required, one that takes in an integer that is the estimated number of words in the text, the other is used for testing purposes. Look at the provided Javadoc. Data Manager – the interface that is provided. The data manager allows the client (user) to create a concordance file or a concordance list (ArrayList of strings). The input is read (from a file or string) and is added to the data structure through the add method. The add method requires a word and a line number. The line number is incremented every time a newline appears in the file or the string.

Answers: 3
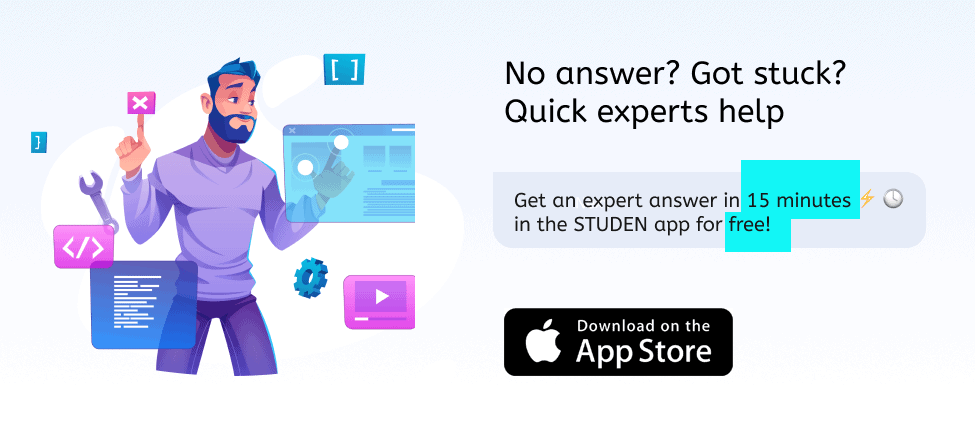

Another question on Computers and Technology

Computers and Technology, 21.06.2019 20:20
Wireless communications is likely to be viewed as an essential part of an enterprise network infrastructure when: select one: a. mobile communication is needed b. communication facilities must be installed at low initial cost c. communication must take place in a hostile or difficult terrain that makes wired communication difficult or impossible d. the same information must be broadcast to many locations
Answers: 1

Computers and Technology, 22.06.2019 05:30
Gerard is currently working as an entry-level customer support technician, but he would like to someday become a software developer. what is the best first step to understand what he should do? ask his manager for a new job or at least a job recommendation study graphic design in order to obtain the necessary skills use career resources to investigate what skills and education are required work part-time as an entry-level web developer question 13 (true/false worth 6 points) (08.03 lc) career resources are used to explore career options and find career information. true false question 14(multiple choice worth 6 points) (08.01 mc) classify the following skills: writing html code, evaluating color theory, using design principles. hard skills interpersonal skills people skills soft skills question 15 (true/false worth 6 points) (08.03 lc) a mentor is a person who is advised, trained, or counseled by a trusted mentee. true false
Answers: 2

Computers and Technology, 22.06.2019 07:50
In this lab, you complete a prewritten c++ program for a carpenter who creates personalized house signs. the program is supposed to compute the price of any sign a customer orders, based on the following facts: the charge for all signs is a minimum of $35.00. the first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. if the sign is made of oak, add $20.00. no charge is added for pine. black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. instructions ensure the file named housesign.cppis open in the code editor. you need to declare variables for the following, and initialize them where specified: a variable for the cost of the sign initialized to 0.00 (charge). a variable for the number of characters initialized to 8 (numchars). a variable for the color of the characters initialized to "gold" (color). a variable for the wood type initialized to "oak" (woodtype). write the rest of the program using assignment statements and ifstatements as appropriate. the output statements are written for you. execute the program by clicking the run button. your output should be: the charge for this sign is $82. this is the code, // housesign.cpp - this program calculates prices for custom made signs. #include #include using namespace std; int main() { // this is the work done in the housekeeping() function // declare and initialize variables here // charge for this sign // color of characters in sign // number of characters in sign // type of wood // this is the work done in the detailloop() function // write assignment and if statements here // this is the work done in the endofjob() function // output charge for this sign cout < < "the charge for this sign is $" < < charge < < endl; return(0); }
Answers: 1

Computers and Technology, 22.06.2019 20:40
Write a program that begins by reading in a series of positive integers on a single line of input and then computes and prints the product of those integers. integers are accepted and multiplied until the user enters an integer less than 1. this final number is not part of the product. then, the program prints the product. if the first entered number is negative or 0, the program must print “bad input.” and terminate immediately. next, the program determines and prints the prime factorization of the product, listing the factors in increasing order. if a prime number is not a factor of the product, then it
Answers: 2
You know the right answer?
Concepts tested by this program Hash Table, Link List, hash code, buckets/chaining, exception handli...
Questions

Social Studies, 27.03.2020 21:00

English, 27.03.2020 21:00

Mathematics, 27.03.2020 21:00


Mathematics, 27.03.2020 21:00

Biology, 27.03.2020 21:00



History, 27.03.2020 21:00

Physics, 27.03.2020 21:00

Mathematics, 27.03.2020 21:00

Mathematics, 27.03.2020 21:00

Mathematics, 27.03.2020 21:00

History, 27.03.2020 21:00




Health, 27.03.2020 21:00


Mathematics, 27.03.2020 21:00