
Computers and Technology, 11.04.2020 03:03 maddiemalmstrom
This programming assignment will consist of a C++ program. Your program must compile correctly and produce the specified output.
Please note that your programs should comply with the commenting and formatting described in the Required Program Development Best Practices document that has been discussed in class and is posted to eLearning. Please see this descriptive file on eLearning for more detailsFor purposes of discussion, let’s specify that inputted number as num.
This program asks you to determine a set of prime numbers, given some input from the user. Your program should ask the user to enter an integer between the range of 1 and 100 inclusive.
If num is outside that range, your program should display an error message and re-prompt (ask) for another number (i. e., have an input validation loop, that has been lectured on in class, described in the book and used in previous program assignments).
Your program should then calculate the set of prime numbers less than and equal to num and display this set of prime numbers to the screen and to an output file named PrimeOut. txt.
All your output, both displayed and to the file, must be neatly formatted, 10 numbers per line, and must look like the following examples.
Use a 5-character output field size for the same input and output manipulator for the output display and out file output to the text file. Since the largest candidate prime in a range for the prime set is a three-digit number (100), this should work well.
For the first example, let’s assume the inputted num is a 20.
The display and the output text file must look like:
The primes that are <= 20 are:
2 3 5 711131719
or if the inputted num is 100:
The primes that are <= 100 are:
2 3 5 7111317192329 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
To solve this problem, your program should have a function called isPrime() that takes an integer as an argument and returns true if the argument is prime or false otherwise. This is an example of a boolean returning function as described in the book in Section 6.9. Note that
taking in a number and determining if it is prime is all this function should do. It should contain no displaying or output to a file statements at all.
The prototype for this function is:
bool isPrime (unsigned number);
Using a function like this can significantly simplify the processing loop in the main program.
It is an example of functional decomposition, also called modular programming. In modular programming, we move the solution of a specific task (in this case: determining if an individual number is prime or not) to a function and then use that function to create the overall solution to our problem.
Functional decomposition is an excellent programming technique and should be used in all of your programs from now on. (Note: for another example of a boolean returning function and how to use it, see p.333 of the book. There the boolean returning function is isEven(int), and you’ll note that it is called inside an "if" statement. You can use your isPrime(int) function in the same way.)
For this problem, it is particularly important to develop your pseudocode before you start programming. Your pseudocode for the main() function might begin as follows:
Open file PrimeOut. txt and verify that it was opened correctly.
Get an unsigned max range number from the user
Verify that number is between 1 and 100 inclusive
for( unsigned number starts at 2; number less than or equal to max range ;
increment number)
If number is prime (call function here)
display number and output number to file End if
End for
Close PrimeOut. txt
You should develop pseudocode for the isPrime(int) function as well. bool isPrime(unsigned number)
{
for (unsigned i starts at 2; i less than or equal to number divided by 2 ; increment i){ if (number modulo i equals 0) {
return false; }
}
return true;

Answers: 1
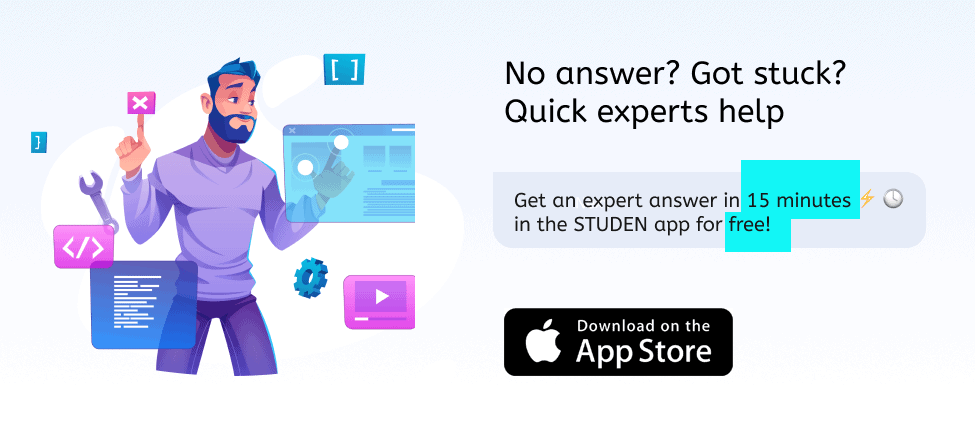

Another question on Computers and Technology

Computers and Technology, 22.06.2019 00:00
11. is the ability to understand how another person is feeling. a. authority b. sympathy c. empathy d. taking a stand
Answers: 1

Computers and Technology, 22.06.2019 13:10
Calculating the "total price" of an item is tedious, so implement a get_item_cost method that just returns the quantity times the price for an item. by the way, the technical term for this kind of instance method is an accessor method, but you'll hear developers calling them getters because they always start with "get" and they get some value from instance attributes. in order to make the items sortable by their total total price, we need to customize our class. search the lectures slides for "magic" to see how to do this. see section 9.8 for an additional reference. the receipt class: this will be the class that defines our receipt type. obviously, a receipt will consist of the items on the receipt. this is called the composition design pattern. and it is very powerful. instance attributes: customer_name : it is very important to always know everything you can about your customers for "analytics", so you will keep track of a string customer name in objects of type receipt. date : the legal team has required that you keep track of the dates that purchases happen for "legal reasons", so you will also keep track of the string date in objects of type receipt. cart_items : this will be a list of the items in the cart and hence end up on the receipt. methods: 1. create a default constructor that can take a customer name as an argument, but if it gets no customer name, it will just put "real human" for the customer_name attribute. it should also accept a date argument, but will just use the value "today" for the date instance attribute if no date is given. the parameters should be named the same as the instance attributes to keep things simple. 2. add_item : self-descriptive. takes a parameter which we hope beyond hope is of type itemtopurchase and adds it to the cart_items. returns none. 3. print_receipt : takes a single parameter isevil, with default value true. returns a total cost of all the items on the receipt (remember to factor in the quantity). prints the receipt based on the following specification: for example, if isevil is true, and customer_name and date are the default values: welcome to evilmart, real human today have an evil day! otherwise, it should print: welcome to goodgo, real human today have an good day! then the receipt should be printed in sorted order like we discussed earlier, but whether or not it starts with the highest cost (think reverse), depends on the value of isevil. if it is evil, then the lowest cost items should print first, but if it is good, then it will print the highest cost items first. (cost meaning price*quantity). remember to return the total cost regardless! your main() function: the main flow of control of your program should go in a main() function or the program will fail all the unit tests. get the name of the customer with the prompt: enter customer name: get the date with the prompt: enter today's date then, ask the question: are you evil? your program should consider the following as true: yeah yup let's face it: yes hint: what do these strings all have in common? your program should consider all the following as false: no nah perhaps but i'm leaning no (just be glad you don't have to handle "yeah no.") okay enough horsing around. (get it? aggies? ! horsing! ) next, in the main() function, you will have to create a receipt object and start adding things into it using an input-while loop. the loop will prompt the user for the item name exactly as in the previous zylab (9.11). but unlike the previous zylab, the loop will terminate only if an empty string is entered for the item name. then, the price and the quantity will be prompted for exactly as in the previous zylab. create the itemtopurchase objects in the same manner as the previous zylab, but don't forget to add them to the receipt using your add_item instance method. then, the items on the receipt should be printed with the same formatting as in the previous zylab, of course with either "good" or "evil" ordering. however, on the last line, the total should be printed as follows: where 10 is replaced by the actual total. sample run here is what a sample run of the final program should look like: enter customer name: nate enter today's date: 12/20/2019 are you evil? bwahahahaha yes enter the item name: bottled student tears enter the item price: 2 enter the item quantity: 299 enter the item name: salt enter the item price: 2 enter the item quantity: 1 enter the item name: welcome to evilmart, nate 12/20/2019 have an evil day! salt 1 @ $2 = $2 bottled student tears 299 @ $2 = $598 total: $600
Answers: 1

Computers and Technology, 23.06.2019 20:30
What are some settings you can control when formatting columns?
Answers: 1

Computers and Technology, 24.06.2019 16:50
Develop the program incrementally: a) start by reading and displaying each line of the input file to make sure you are reading the data set correctly. b) use the split string method to extract information from each line into a list. print the list to prove that this step is working correctly. d) convert the exam scores to type int and calculate the student’s average. display those items to prove this step is working correctly. e) create a tuple containing the six items for each student (name, exam scores, exam mean). display the tuples to prove this step is working correctly. (optionally, you may want to have the exam scores in a list so your tuple is (name, scores_list, f) append each tuple to a list. display the list to prove this step is working correctly. g) use the sort list method to re-order the tuples in the list. display the list to prove this step is working correctly. h) use a for statement to display the contents of the list as a table (with appropriate formatting). i) use a for statement to calculate the average of all scores on exam #1, then display the results. note that you could have calculated this average within the first loop, but we are explicitly requiring you to do this calculation by looping though your list of tuples. j) add the logic to calculate the average of all scores on exam #2, then display the results.
Answers: 2
You know the right answer?
This programming assignment will consist of a C++ program. Your program must compile correctly and p...
Questions

Mathematics, 23.05.2020 08:00



















Computers and Technology, 23.05.2020 08:00