
Computers and Technology, 12.03.2020 23:47 jaylenmiller437
Scenario: You are to build a Java application that will implement the daily business of a small loan company, Midwest Loan. This company makes loans to individuals and allows those individuals to pay off those loans with monthly payments. They offer low-interest rates but do not allow early loan re-payment (i. e., each month’s loan payment will be the same).
Overall Program Usage Requirements:
• Your program will use a Menu system. The user will be a Loan company employee (you will not need a login, etc.). Using the menu system, the employee should be able to do the following major tasks all from a main menu:
1) Add a loan customer. This is a new customer who has taken out a new loan. Your application should be able to create as many new customers as the employee using the system wants.
2) Print the loan amortization table for any user stored in the system. Once this menu item is selected, the employee will see a list of all customers, then be prompted to enter a UserID to print the table for.
3) Exit the application.
Detailed requirements for each menu option:
Adding a Loan Customer:
• Your application should allow the employee to create as many new customers as needed. Remember that loan customers will NOT be using this system, only employees.
• Each new customer should have a unique ID number. Use an int to accomplish this.
• You will store the customer ID number, first name, last name, address, cell phone number, and age in a two-dim String array.
• You will store the customer ID number, loan amount, and loan interest rate (as a #.# number), and desired monthly payment amount in a two-dim double array.
o Notice that the idea here is that these two arrays "sync" up. The first customer in the information array corresponds to the first customer in the loan info array, and etc. HINT: Matching the String version of the customer ID and the double version in the loan array will take a little thought!
• You will have to collect this information from the console when the employee chooses to create a new customer.
Print the loan amortization table for any user stored in the system:
• You will print to the screen the customer IDs and Customer Name of each customer stored in the system if this menu option is used. The employee can then type in a number and hit enter, and the full loan amortization table will print for that customer. The application will then return to the main menu.
• After the employee enters a customer ID your code should behave in the following order:
1) First, you will invoke a method that you will create called loanLength( ). This loan will return back an int representing the number of months of the life of the loan. For example, some loans principals and monthly payments mean they will be done in 60 months, some in 72 months, etc. Your method will loop and simulate the loan and the monthly payments, counting the months until the balance reaches 0, then returns back that number of months of the life of the loan. You will need to pass some data to the method through its parameters for the user chosen so it can do this! I will let you decide what and how this data is passed.
2) Once you have the "life" of the loan in the number of months, create a new two-dim array who number of rows is the number of months in the loan. Populate/fill the columns of the two-dim array with each month’s information: Balance, interest on that balance for that month, monthly payment, new balance. The company calculates the new ending balance for that month as:
(current balance + interest accumulated) – monthly payment You are basically saving the amortization table into the two-dim array!
3) Once you have the two-dim array populated, pass it as a reference to a method called printAmortTable( ). The method will have a void return type. The method’s purpose is to print a professional looking amortization table using the information in the two-dim double array you pass to the method through its parameter. Use your .printf().
4) Once the overall printing is done, the program returns to the main menu.

Answers: 3
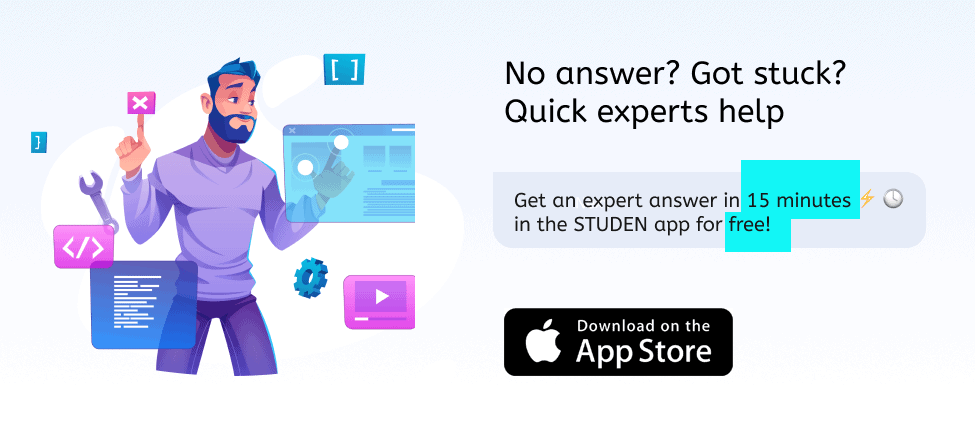

Another question on Computers and Technology

Computers and Technology, 22.06.2019 15:00
This is not a factor that you should use to determine the content of your presentation. your audience your goals your purpose your technology
Answers: 1

Computers and Technology, 22.06.2019 18:30
Which of the following commands is more recommended while creating a bot?
Answers: 1

Computers and Technology, 23.06.2019 02:50
There’s only one game mode that stars with the letter ‘e’ in cs: go. which of the options below is it?
Answers: 1

Computers and Technology, 23.06.2019 09:20
How to print: number is equal to: 1 and it is odd number number is equal to: 2 and it is even number number is equal to: 3 and it is odd number number is equal to: 4 and it is even number in the console using java using 1 if statement, 1 while loop, 1 else loop also using % to check odds and evens
Answers: 3
You know the right answer?
Scenario: You are to build a Java application that will implement the daily business of a small loan...
Questions

Biology, 02.02.2020 10:44

Chemistry, 02.02.2020 10:44





Mathematics, 02.02.2020 10:44

Mathematics, 02.02.2020 10:44

Biology, 02.02.2020 10:44

Geography, 02.02.2020 10:44

Biology, 02.02.2020 10:44

Mathematics, 02.02.2020 10:44


Mathematics, 02.02.2020 10:44


Advanced Placement (AP), 02.02.2020 10:45

Mathematics, 02.02.2020 10:45

Mathematics, 02.02.2020 10:45

History, 02.02.2020 10:45

French, 02.02.2020 10:45