
Computers and Technology, 21.02.2020 20:36 LordBooming
In this assignment, you will make another calculator. This one will work on arrays rather
than single values. Like the basic calculator from the previous assignments, the array
calculator should allow users to add, subtract, multiply and divide the corresponding
values in two arrays. It should also allow users to generate a random array. Finally,
there is a special value that can be computed for two arrays called the dot product that
your calculator should also be able to compute. You can find the definition of the
algebraic dot product of two arrays by googling (Wikipedia is a good choice). Briefly, the
dot product of two arrays is the sum of the product of the corresponding values in the
arrays.
For example, the dot product of [1, 5, 8, 2] and [2, 4, 1, 3] is:
(1 * 2) + (5 * 4) + (8 * 1) + (2 * 3)
which is 36.
Your array should have the same methods as the calculator from Assignment 4, plus a
method to compute the dot product. These methods should deal with double arrays
rather than plain doubles, however. Here is a list of the method signatures:
public static int getMenuOption()
public static double[] getOperand(String prompt, int size);
public static double getOperand(String prompt);
public static double[] add(double[] operand1, double[] operand2)
public static double[] subtract(double[] operand1, double[] operand2)
public static double[] multiply(double[] operand1, double[] operand2)
public static double[] divide(double[] operand1, double[] operand2)
public static double[] random(double lowerLimit, double upperLimit, int size)
public static double dotProduct(double[] operand1, double[] operand2)
You will need two versions of the getOperand method. This is an example of method
overloading. The first version will prompt the user for enough values to fill an array and
return the array. This will be used to get the operands for the add, subtract, multiply,
divide, and dotProduct method. The other version does the same thing as in the Fourth
Assignment β Calculator with Methods β it displays a prompt and reads and returns a
double value. This is needed to get the inputs for the random method. The random
method will need one additional parameter β the size of the random array that should be
generated. Note that the dotProduct method only needs to return a single double value
rather than an array.
Here is an example run of the program:
Menu
1. Add
2. Subtract
3. Multiply
4. Divide
5. Dot product
6. Generate random array
7. Quit
What would you like to do? 1
How many values are in the arrays? 3
Enter the values in the first array, separated by spaces:
2 4 6
Enter the values in the second array, separated by spaces:
1 3 5
The result is [3.0, 7.0, 11.0]

Answers: 3
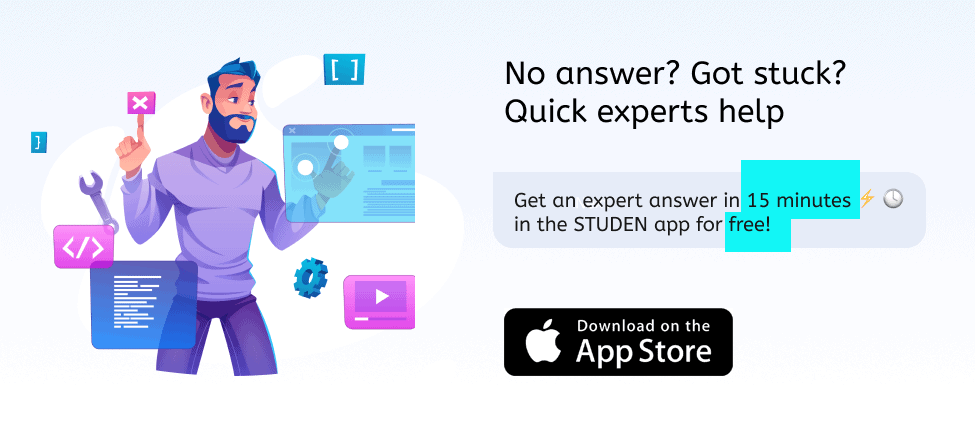

Another question on Computers and Technology

Computers and Technology, 23.06.2019 12:00
If you embed a word table into powerpoint, what happens when you make edits to the embedded data? a. edits made to embedded data change the data in the source file; however, edits made to the source file will not be reflected in the embedded data. b. edits made to embedded data will change the data in the source file, and edits made to the source file will be reflected in the embedded data. c. edits made to embedded data don't change the data in the source file, nor will edits made to the source file be reflected in the embedded data. d. edits made to embedded data don't change the data in the source file; however, edits made to the source file will be reflected in the embedded data.
Answers: 1

Computers and Technology, 23.06.2019 18:30
Write a program that prints the day number of the year, given the date in the form month-day-year. for example, if the input is 1-1-2006, the day number is 1; if the input is 12-25-2006, the day number is 359. the program should check for a leap year. a year is a leap year if it is divisible by 4, but not divisible by 100. for example, 1992 and 2008 are divisible by 4, but not by 100. a year that is divisible by 100 is a leap year if it is also divisible by 400. for example, 1600 and 2000 are divisible by 400. however, 1800 is not a leap year because 1800 is not divisible by 400.
Answers: 3

Computers and Technology, 24.06.2019 07:30
Consider the folloeing website url: what does the "http: //" represent? a. protocal identifier. b. ftp. c. domain name d. resource name
Answers: 2

You know the right answer?
In this assignment, you will make another calculator. This one will work on arrays rather
Questions



Mathematics, 16.11.2020 23:40

Mathematics, 16.11.2020 23:40

Mathematics, 16.11.2020 23:40

Arts, 16.11.2020 23:40


Biology, 16.11.2020 23:40

History, 16.11.2020 23:40

History, 16.11.2020 23:40

Mathematics, 16.11.2020 23:40






English, 16.11.2020 23:40

Computers and Technology, 16.11.2020 23:40
