java programming
9.9 ch 9, part 2: arraylist searching
import java. util. a...

Computers and Technology, 18.11.2019 23:31 grangian06
java programming
9.9 ch 9, part 2: arraylist searching
import java. util. arraylist;
public class arraylistset {
/**
* searches through the arraylist arr, from the first index to the last, returning an arraylist
* containing all the indexes of strings in arr that match string s. for this method, two strings,
* p and q, match when p. equals(q) returns true or if both of the compared references are null.
*
* @param s the string to search for.
* @param arr the arraylist of string to search.
* @return an arraylist of the indexes in arr that match s. the arraylist will be ordered from
* the smallest index to the largest. if arr is null, the method will return null.
*/
public static arraylist findexact(string s, arraylist arr) {
return null;
}
/**
* searches through the arraylist arr, from the first index to the last, returning an arraylist
* containing all the indexes of strings in arr that contains string s. for this method, a
* strings p contains q when p. contains(q) returns true or if both of the compared references are null.
*
* @param s the string to search for.
* @param arr the arraylist of string to search.
* @return an arraylist of the indexes in arr that contains s. the arraylist will be ordered from
* the smallest index to the largest. if arr is null, the method will return null.
*/
public static arraylist findcontains(string s, arraylist arr) {
return null;
}
/**
* adds string s to the end of the arraylist arr if and only if arr does not already have an
* element that matches s.
*
* do not duplicate code! use your findexact method to check if arr contains s.
*
* @param s the string to add (if not already in arr).
* @param arr the arraylist in which to s (if a string with the same contents is not already in
* arr).
* @return the number of times that s is found in arr after attempting to adding s. if arr is null,
* return -1.
*/
public static int adduniquestring(string s, arraylist arr) {
return -42;
}
}
import java. util. arraylist;
import java. util. arrays;
public class testarraylistset {
public static boolean testfindexact() {
system. out. println("starting ");
//test 1
{
arraylist ret =
arraylistset. findexact("foo", new arraylist(arrays. aslist("foo","bar","foo";
arraylist exp = new arraylist(arrays. aslist(0, 2));
if(! ret. equals(exp)) {
system. out. println("test 1 failed! ");
system. out. println("returned: " + ret);
system. out. println("expected: " + exp);
return false;
}
}
system. out. println(" testfindexact");
return true;
}
public static boolean testfindcontains() {
system. out. println("starting ");
//test 1
{
arraylist ret =
arraylistset. findcontains("o", new arraylist(arrays. aslist("foo","bar","foo";
arraylist exp = new arraylist(arrays. aslist(0, 2));
if(! ret. equals(exp)) {
system. out. println("test 1 failed! ");
system. out. println("returned: " + ret);
system. out. println("expected: " + exp);
return false;
}
}
system. out. println(" testfindcontains");
return true;
}
public static boolean testadduniquestring() {
system. out. println("starting ");
//test 1
{
arraylist arr = new arraylist(arrays. aslist("a","b","c"));
arraylist exp = new arraylist(arr);
exp. add("d");
int retindex = arraylistset. adduniquestring("d", arr);
int expindex = 1;
if(! arr. equals(exp) || retindex ! = expindex) {
system. out. println("test 1 failed! ");
system. out. println("returned value: " + retindex + "; expected " + expindex);
system. out. println("list after: " + arr);
system. out. println("expected: " + exp);
return false;
}
}
//test 2
{
arraylist arr = new arraylist(arrays. aslist("a","b","b","c"));
arraylist exp = new arraylist(arr);
int retindex = arraylistset. adduniquestring("b", arr);
int expindex = 2;
if(! arr. equals(exp) || retindex ! = expindex) {
system. out. println("test 2 failed! ");
system. out. println("returned value: " + retindex + "; expected " + expindex);
system. out. println("list after: " + arr);
system. out. println("expected: " + exp);
return false;
}
}
system. out. println(" testadduniquestring");
return true;
}
public static void main(string[] args) {
testfindexact();
testfindcontains();
testadduniquestring();
}
}

Answers: 2
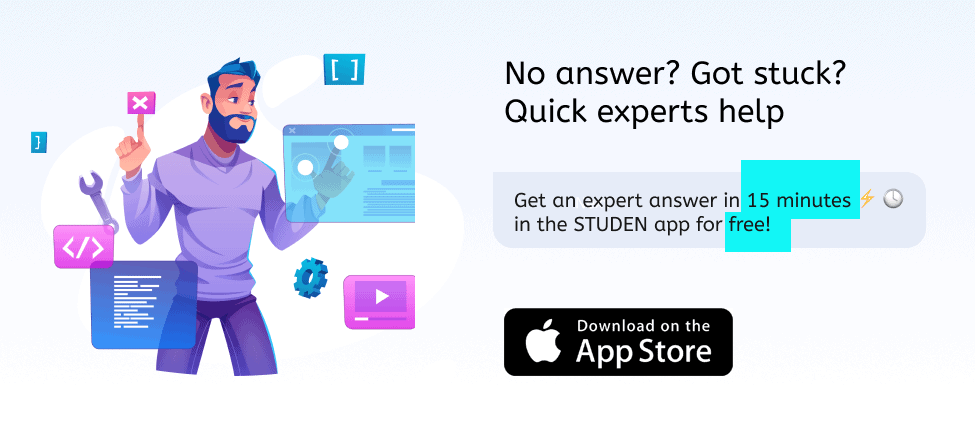

Another question on Computers and Technology

Computers and Technology, 21.06.2019 17:30
How many pairs of chromosomes do human body cells contain?
Answers: 2

Computers and Technology, 22.06.2019 23:00
Suppose s, t, and w are strings that have already been created inside main. write a statement or statements, to be added to main, that will determine if the lengths of the three strings are in order by length, smallest to largest. that is, your code should determine if s is strictly shorter than t, and if t is strictly shorter than w. if these conditions hold your code should print (the boolean value) true. if not, your code should print false. (strictly means: no ties) example: if s, t, and w are "cat", "hats", and "skies" your code should print true - their lengths are 3-4-5; but if s, t, and w are "cats" "shirt", and "trust", then print false - their lengths are 4-5-5 enter your code in the box below
Answers: 2

Computers and Technology, 22.06.2019 23:50
List a few alternative options and input and output over the standerd keyboard and monitor. explain their functioning in details.
Answers: 2

Computers and Technology, 23.06.2019 19:00
Whose task it is to ensure that the product flows logically from one step to another?
Answers: 3
You know the right answer?
Questions


English, 01.07.2019 14:20



Chemistry, 01.07.2019 14:20



Arts, 01.07.2019 14:20


Chemistry, 01.07.2019 14:20

Chemistry, 01.07.2019 14:20

Chemistry, 01.07.2019 14:20

Physics, 01.07.2019 14:20


History, 01.07.2019 14:20





Biology, 01.07.2019 14:20