
Computers and Technology, 11.07.2019 21:30 jet0120996
You have the templates of 2 classes, person and program. the person class has 4 attributes, name, age, major and gpa. there is a constructor for the class that is used to make person objects. there are setter/mutator methods and getter/accessor methods for those attributes. there is also a printinfo() method that prints out information about the person. the program class has a main method that has 5 person objects person1 to person5 declared and initialized. the main method within the class also has a scanner object that is used to take input for a person number and a newgpa. your task is as follows:
a. based on the input of personselect select the appropriate person and change their gpa to the newgpa input and then print out their information. for instance if the user types in 1 then select person 1 and print out their information using the printinfo method. do it for all possible inputs from 1 to 5.
b. if however the input is not 1 to 5 then inform the user they have to have to type in an appropriate person number
person. java
public class person {
string name;
int age;
double gpa;
string major;
public person(string aname, int aage, string amajor, double agpa)
{
name = aname;
age = aage;
gpa = agpa;
major = amajor;
}
/**
* @return the name
*/
public string getname() {
return name;
}
/**
* @param name the name to set
*/
public void setname(string pname) {
name = pname;
}
/**
* @return the age
*/
public int getage() {
return age;
}
/**
* @param age the age to set
*/
public void setage(int page) {
age = page;
}
/**
* @return the gpa
*/
public double getgpa() {
return gpa;
}
/**
* @param gpa the gpa to set
*/
public void setgpa(double pgpa) {
gpa = pgpa;
}
/**
* @return the major
*/
public string getmajor() {
return major;
}
/**
* @param major the major to set
*/
public void setmajor(string pmajor) {
major = pmajor;
}
public void printinfo()
{
system. out. println("");
system. out. println("business college ");
system. out. println("name: " + name);
system. out. println("major: " + major);
system. out. println("gpa: " + gpa);
system. out. println("");
}
}
program.
import java. util. scanner;
public class program {
public static void main(string[] args) {
//declare person objects here
person person1 = new person("dewars",40 , "mis", 3.9);
person person2 = new person("budweiser",23 , "mis", 3.2);
person person3 = new person("appletons",25 , "mis", 3.0);
person person4 = new person("beam",20 , "finance", 3.7);
person person5 = new person("daniels",19 , "accounting", 2.9);
scanner scan = new scanner(system. in);
system. out. println("type in a person number whose gpa you would like to change > ");
int personselect = scan. nextint();
system. out. println("type in the gpa you would like the person's to be > ");
double newgpa = scan. nextdouble();
/*
* code here
*/
}
}

Answers: 1
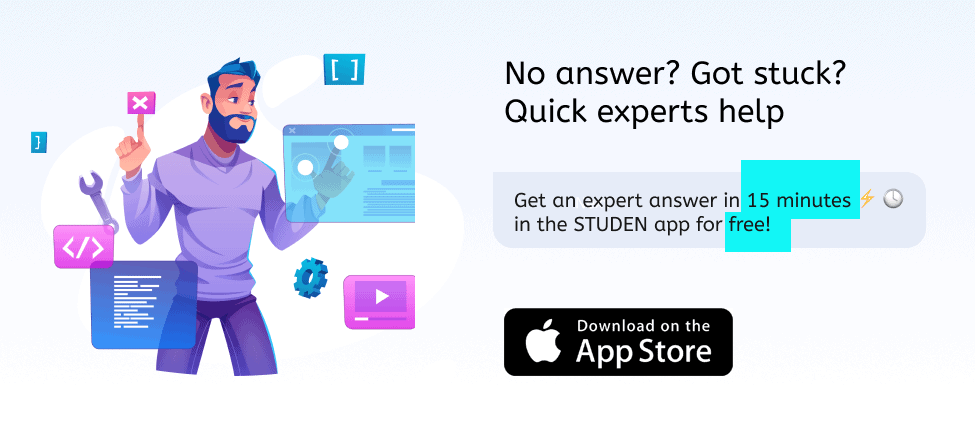

Another question on Computers and Technology

Computers and Technology, 22.06.2019 10:20
Shown below is the start of a coding region within the fist exon of a gene. 5'--3' 3'--5' how many cas9 pam sequences are present?
Answers: 1

Computers and Technology, 22.06.2019 12:40
In a response of approximately 50 words, explain why it would be essential for the successful a/v technician to participate in additional coursework, presentations and seminars offered by equipment manufacturers as well as annual conferences attended by colleagues in the industry.
Answers: 1

Computers and Technology, 23.06.2019 18:00
What can a word user do with the customize ribbon dialog box? check all that apply. minimize the ribbon add a new tab to the ribbon remove a group from a tab add a group to a tab choose which styles appear choose which fonts appear choose tools to appear in a group
Answers: 1

Computers and Technology, 23.06.2019 19:30
Amitha writes up a one-page summary of a novel during her summer internship at a publishing company. when she reads over the page, she realizes she used the word “foreshadow” seven times, and she would like to reduce the repetition. which tool would best amitha solve this problem?
Answers: 3
You know the right answer?
You have the templates of 2 classes, person and program. the person class has 4 attributes, name, ag...
Questions

Chemistry, 20.09.2020 15:01

Social Studies, 20.09.2020 15:01

Mathematics, 20.09.2020 15:01

History, 20.09.2020 15:01


English, 20.09.2020 15:01


Mathematics, 20.09.2020 15:01



English, 20.09.2020 15:01

Mathematics, 20.09.2020 15:01

English, 20.09.2020 15:01


History, 20.09.2020 15:01


History, 20.09.2020 15:01


Mathematics, 20.09.2020 15:01

Mathematics, 20.09.2020 15:01